Max aggregate operator is used to find the largest item from a collection.
Exapmle:-
In the below example we created a complex type collection for an Employee. This Employee collection contains EmpId, EmpName and Age. We need to find a maximum employee age.
Code:-
IList<Employee> empList = new List<Employee>() {
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "John", Age = 33} ,
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "Mohan", Age = 41 } ,
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "Bin", Age = 28 } ,
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "Ram" , Age = 20} ,
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "Rohan" , Age = 35 }
};
var oldestEmp = empList.Max(s => s.Age);
Console.WriteLine("Oldest Employee Age: {0}", oldestEmp);
Output:-
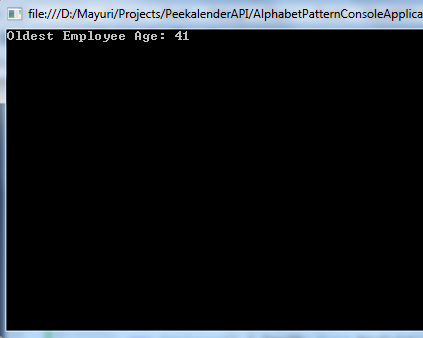
Max aggregate operator is not supported in C# Query syntax but it is supported in VB.Net.
Query syntax in vb.net:-
code:-
Dim empList = New List(Of Employee) From {
New Employee() With {.EmpID = Guid.NewGuid().ToString(), .EmpName = "John", .Age = 13},
New Employee() With {.EmpID = Guid.NewGuid().ToString(), .EmpName = "Mohan", .Age = 21},
New Employee() With {.EmpID = Guid.NewGuid().ToString(), .EmpName = "Bin", .Age = 18},
New Employee() With {.EmpID = Guid.NewGuid().ToString(), .EmpName = "Ram", .Age = 20},
New Employee() With {.EmpID = Guid.NewGuid().ToString(), .EmpName = "Rohan", .Age = 15}
}
Dim empMaxAge = Aggregate emp In empList Into Max(emp.Age)
Console.WriteLine("Maximum Age of Employee: {0}", empMaxAge);
Output:-
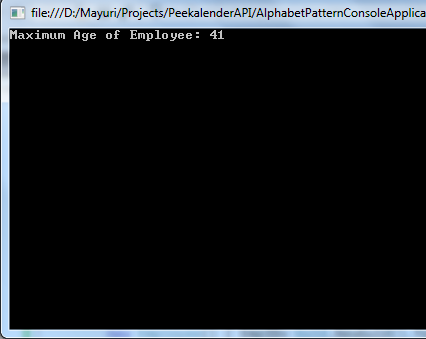
0 Comment(s)