To integrate your iOS app with Google for allowing login from Google, Share and other features we need integration by Google login SDK. So in this tutorial, I am providing you simple steps to integrate Google Login SDK using swift in iOS.
Let's see how:
Step 1. Create a project in Xcode in which you want to integrate Google sign-in SDK. First of all, create a pod file and install pods of Google SDK this can be done easily by given below steps:-
• Open terminal app in the system -> write command cd xyz/… (path of project in system)
pod init (this command use to create a pod file. Open pod file with Xcode you are using)
• Write few lines in created pod file:-
pod 'Google/SignIn'
• Go back to terminal app and write command:-
pod install
• After successful pod installation, you will get message in terminal:-
Pod installation complete! There are1 dependencies from the Podfile and 11 total pods installed.
Step 2. For further processing, you need to register on google developer account (goo.gl/8qXVbF) and after registration, the GoogleServices-Info.plist file is available to download which is required to get client id and URL.
Step 3. Now add the GoogleService-Info.plist to your project and copy reversed_client_id and add in your project in given below way:-
open Xcode project -> target - >info -> url (in url paste copied reversed_client_id)
Step 4. Add the below code in AppDelegate.swift file:-
//
// AppDelegate.swift
// GoogleSignIn
//
// Created by elcaptain1 on 8/8/17.
// Copyright 2017 Tanuja. All rights reserved.
//
import UIKit
import GoogleSignIn
import Google
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
// Override point for customization after application launch.
GIDSignIn.sharedInstance().clientID = "118742319601-6rm67r0h9rn8akm8h6t4t0kv0mc83hdl.apps.googleusercontent.com" // this key you will get in google service info plist i.e client_id
return true
}
func applicationWillResignActive(_ application: UIApplication) {
// Sent when the application is about to move from active to inactive state. This can occur for certain types of temporary interruptions (such as an incoming phone call or SMS message) or when the user quits the application and it begins the transition to the background state.
// Use this method to pause ongoing tasks, disable timers, and invalidate graphics rendering callbacks. Games should use this method to pause the game.
}
func applicationDidEnterBackground(_ application: UIApplication) {
// Use this method to release shared resources, save user data, invalidate timers, and store enough application state information to restore your application to its current state in case it is terminated later.
// If your application supports background execution, this method is called instead of applicationWillTerminate: when the user quits.
}
func applicationWillEnterForeground(_ application: UIApplication) {
// Called as part of the transition from the background to the active state; here you can undo many of the changes made on entering the background.
}
func applicationDidBecomeActive(_ application: UIApplication) {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
}
func applicationWillTerminate(_ application: UIApplication) {
// Called when the application is about to terminate. Save data if appropriate. See also applicationDidEnterBackground:.
}
func application(_ app: UIApplication, open url: URL, options: [UIApplicationOpenURLOptionsKey : Any]) -> Bool {
return GIDSignIn.sharedInstance().handle(url,
sourceApplication: options[UIApplicationOpenURLOptionsKey.sourceApplication] as? String,
annotation: options[UIApplicationOpenURLOptionsKey.annotation])
}
}
Step 5. Now in Main.storyboard for user interface we will take one button on view controller and on button click action we will write code for the google sign in which is given below:-
//
// ViewController.swift
// GoogleSignIn
//
// Created by elcaptain1 on 8/8/17.
// Copyright 2017 Tanuja. All rights reserved.
//
import UIKit
import GoogleSignIn
class ViewController: UIViewController,GIDSignInDelegate,GIDSignInUIDelegate {
override func viewDidLoad() {
super.viewDidLoad()
GIDSignIn.sharedInstance().delegate = self
GIDSignIn.sharedInstance().uiDelegate = self
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func btnGoogleSignn(_ sender: AnyObject) {
GIDSignIn.sharedInstance().signOut()
GIDSignIn.sharedInstance().signIn()
}
func sign(_ signIn: GIDSignIn!, didSignInFor user: GIDGoogleUser!, withError error: Error!) {
if (error == nil) {
//initializeActivity()
let userId = user.userID as NSString
let idToken = user.authentication.idToken
let userName = user.profile.name as NSString
let givenName = user.profile.givenName
let familyName = user.profile.familyName
let email = user.profile.email as NSString
print("Token == \(idToken) givenName == \(givenName!) familyName==\(familyName!) userId == \(userId) userName == \(userName) email == \(email)")
} else {
print("\(error.localizedDescription)")
if((error) != nil)
{
print("DrLoveMatch\n\(error.localizedDescription)")
}
}
}
}
Output:-
After following the above-mentioned steps you can easily integrate Google-sign-in in your application. Output screens for the same are given below:-
1. If some other user is already logged-in then user will get to see that option also along with another user account option as shown in below screenshot:-
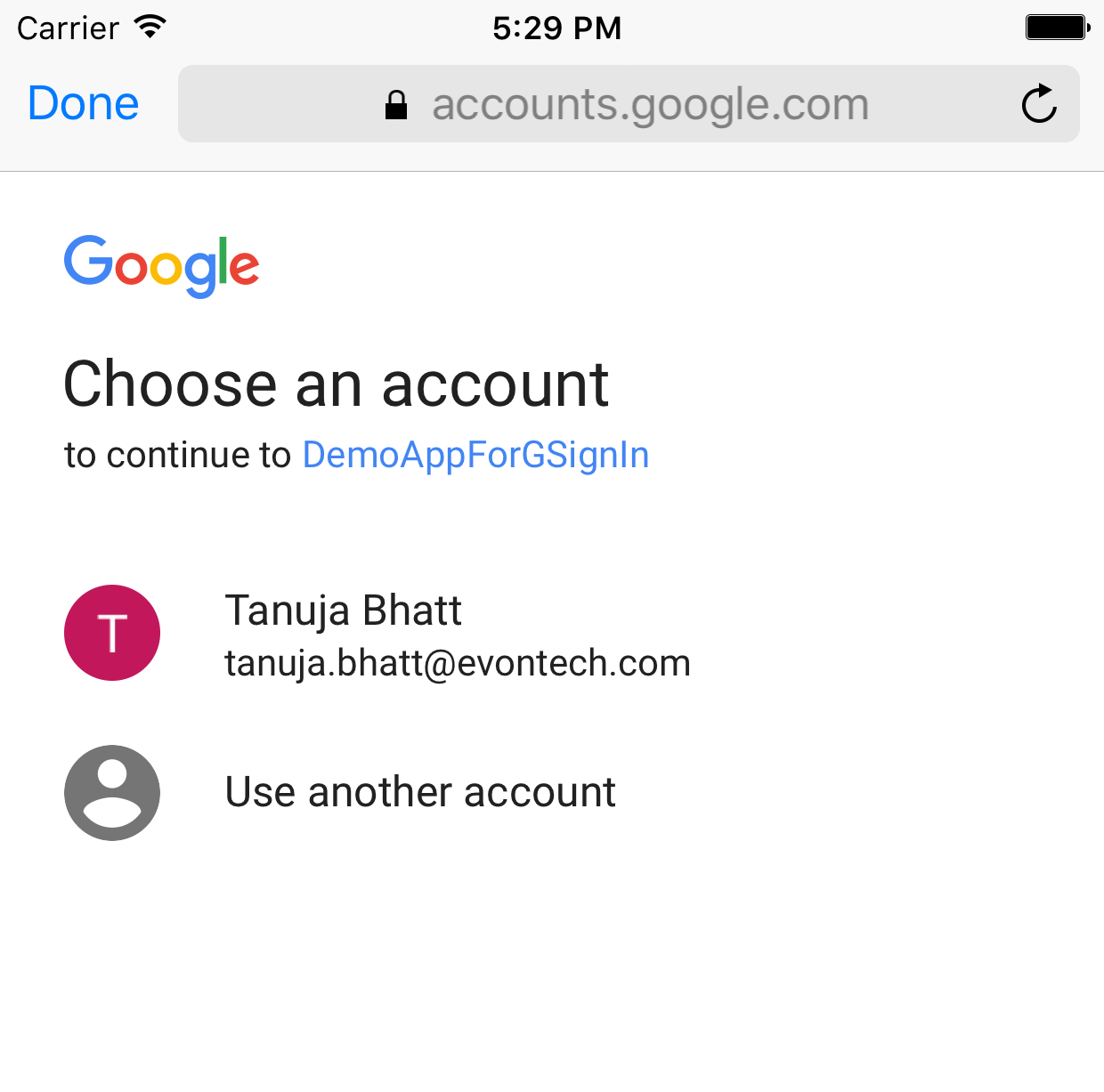
2. If user select another account then this new screen will be shown and user is able to enter new credentials for google login as shown in below screenshot:-
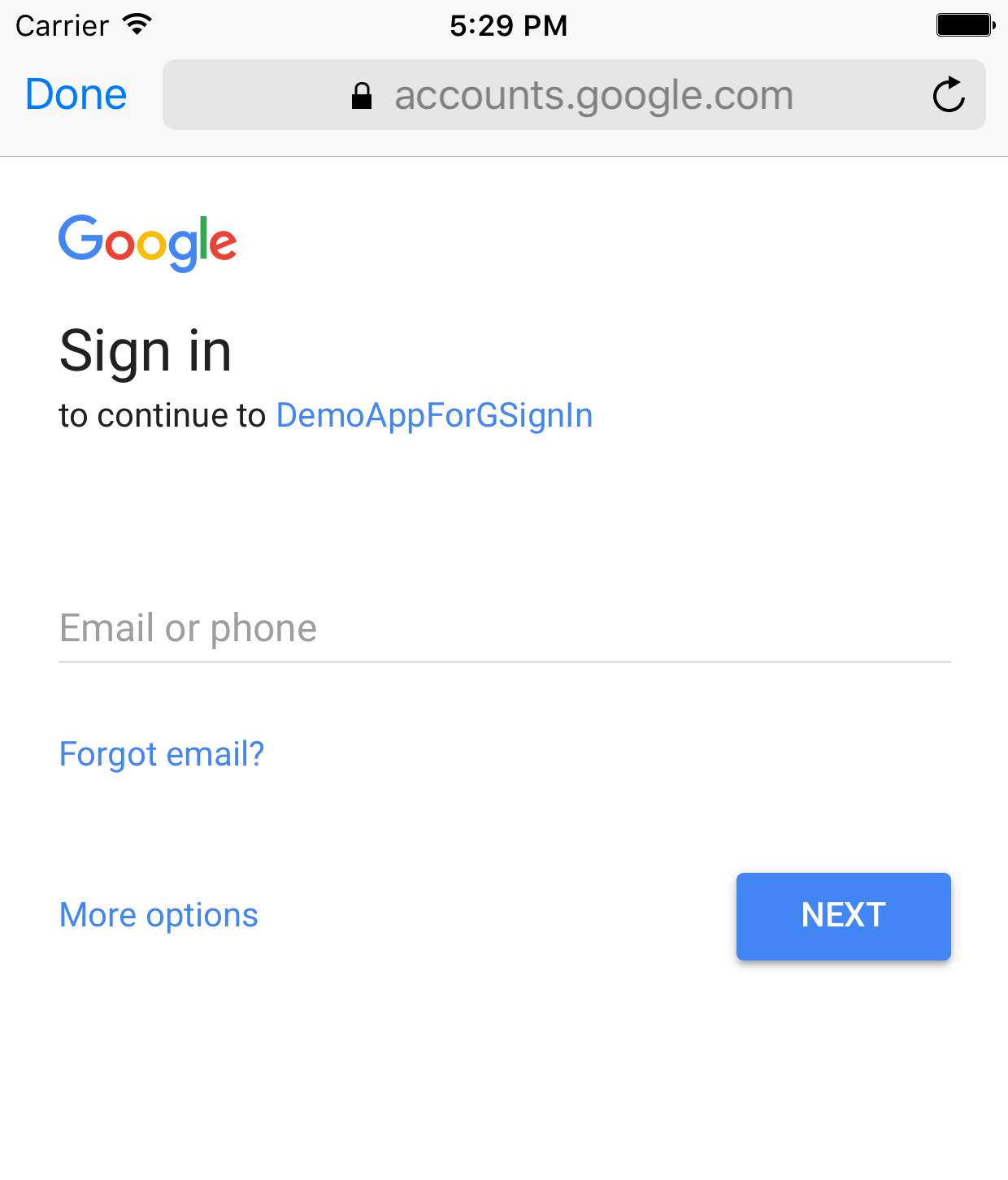
3. After entering email id now the new screen will be open for password entry and after user logged-in, some user information will get the print as in code we are printing some details of the logged-in user.
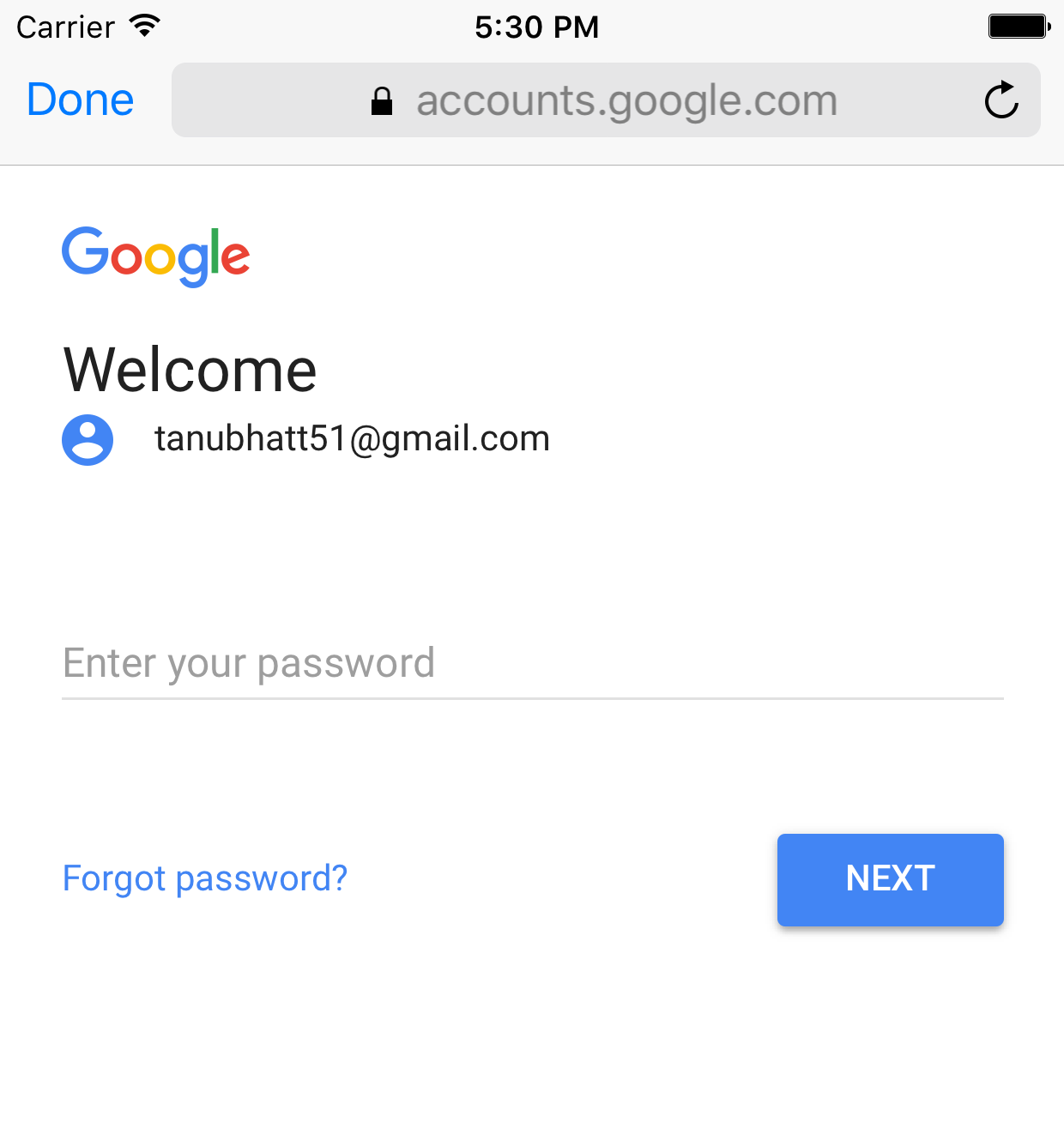
Text output will be shown as :-
givenName == Tanuja familyName == Bhatt userId == 103910906542925056815 userName == Tanuja Bhatt email == tanuja.bhatt@evontech.com
0 Comment(s)