To display or load more results, whether it is commenting on a blog website, like or images on social media or products on eCommerce website the best UX pattern for this is either pagination, a Load more button or infinite scrolling. In this tutorial, we will see an "easy trick to implement load more dynamic results functionality using jQuery, Ajax Php, and Database".
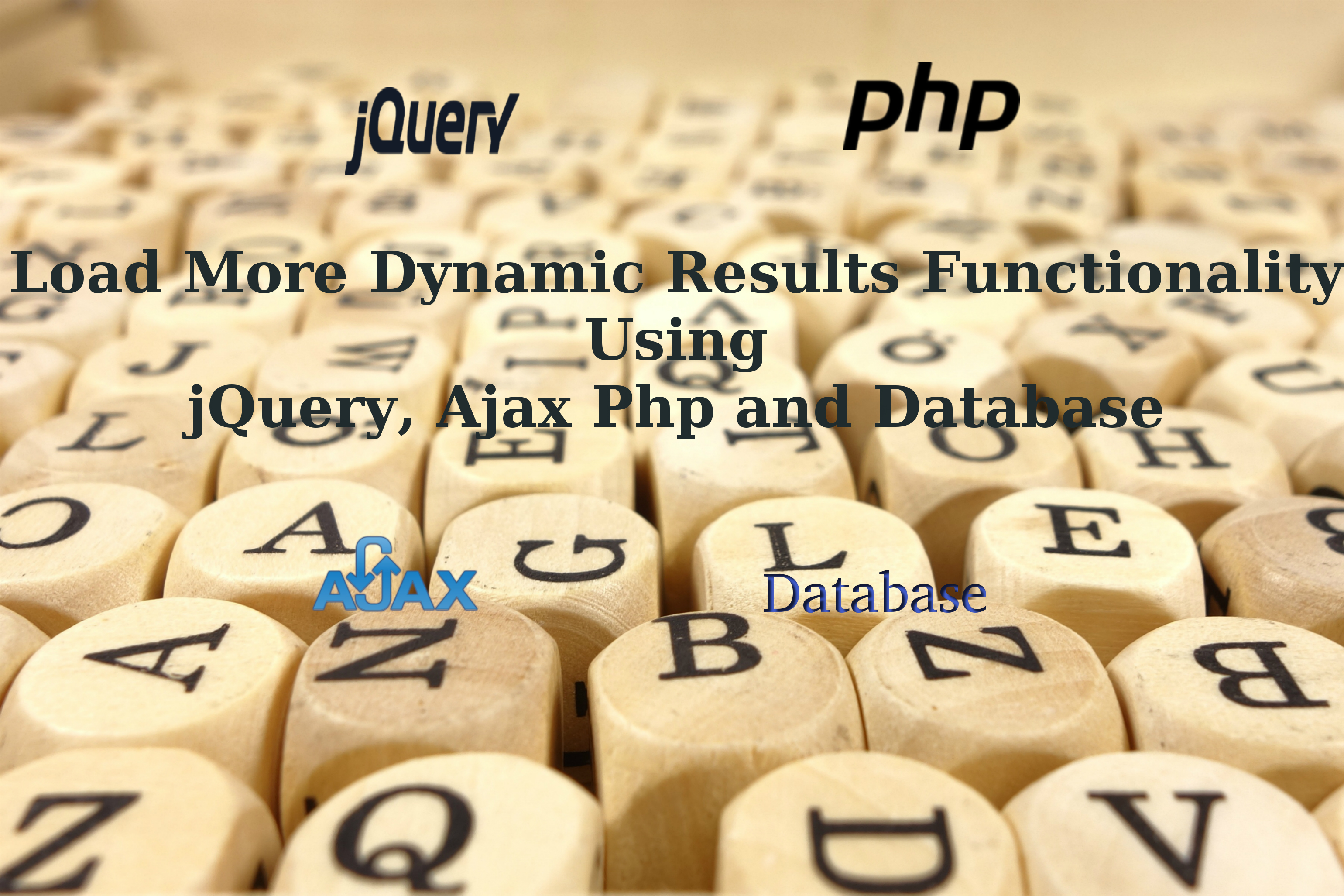
Basically, following tutorial require mainly 3 files to process and display more dynamic results. ( let's assume blog posts as results in our example).
Each time user clicks on 'load more' button, two blog post will display one by one till all the blogs from the table are being fetched.
One file is to hold database connection, Another one containing HTML/PHP/jquery code will request an ajax call on button click and another PHP file will process ajax request and send back the blog details to HTML file.
/* Create Database connection to include in desired files as db_config.php file */
<?php
$db_host = 'localhost';
$user = 'db_user_name';
$pass = 'db_password';
$db_name = 'db_name';
$con = mysqli_connect($db_host, $user, $pass, $db_name);
?>
/* Create main index file to show blog posts with load more functionality.Initially there will be 2 blog post. */
<html lang='en-US' xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Load more data using jQuery, Ajax and PHP </title>
<style type="text/css">
.blog_item {
padding: 1px 12px;
background-color:#F1F1F1;
margin: 5px 15px 2px;
}
.blog_item a {
text-decoration: none;
padding-bottom: 2px;
color: #0074a2;
}
.show_more_container {
margin: 15px 25px;
}
.show_more {
cursor: pointer;
display: block;
padding: 10px 0;
text-align: center;
font-weight:bold;
}
</style>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.2/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function(){
$(document).on('click','.show_more',function(){
var ID = $(this).attr('id');
$('.show_more').hide();
$.ajax({
type:'POST',
url:'ajax_more_posts.php',
data:'pkBlogId='+ID,
success:function(html){
$('#show_more_container'+ID).remove();
$('.blog_list').append(html);
}
});
});
});
</script>
</head>
<body>
<?php
//include database config
include('db_config.php');
?>
<h1 style="text-align: center;">Load more blog items</h1>
<div class="blog_list">
<?php
//get rows query
$query = mysqli_query($con, "SELECT * FROM blogs ORDER BY pkBlogId DESC LIMIT 2");
$numRows = mysqli_num_rows($query);
if($numRows > 0){
while($row = mysqli_fetch_assoc($query)){
$blog_id = $row['pkBlogId']; ?>
<div class="blog_item"><a href="javascript:void(0);"><h2><?php echo $row['blogTitle']; ?></h2></a></div>
<?php } ?>
<div class="show_more_container" id="show_more_container<?php echo $blog_id; ?>">
<span id="<?php echo $blog_id; ?>" class="show_more" title="Load more posts">Show more</span>
</div>
<?php } ?>
</div>
</body>
</html>
/* The following content will be used in ajax_more_posts.php file to request */
<?php
if(isset($_POST["pkBlogId"]) && !empty($_POST["pkBlogId"])){
//include database config
include('db_config.php');
$queryAll = mysqli_query($con,"SELECT COUNT(*) as num_rows FROM blogs WHERE pkBlogId < ".$_POST['pkBlogId']." ORDER BY pkBlogId DESC");
$row = mysqli_fetch_assoc($queryAll);
//count all rows except already displayed
$allRows = $row['num_rows'];
$showLimit = 2;
//get rows query
$query = mysqli_query($con, "SELECT * FROM blogs WHERE pkBlogId < ".$_POST['pkBlogId']." ORDER BY pkBlogId DESC LIMIT ".$showLimit);
$numRows = mysqli_num_rows($query);
if($numRows > 0){
while($row = mysqli_fetch_assoc($query)){
$blog_id = $row["pkBlogId"]; ?>
<div class="blog_item"><a href="javascript:void(0);"><h2><?php echo $row["blogTitle"]; ?></h2></a></div>
<?php } ?>
<?php if($allRows > $showLimit){ ?>
<div class="show_more_container" id="show_more_container<?php echo $blog_id; ?>">
<a id="<?php echo $blog_id; ?>" class="show_more" title="Load more posts">Show more</a>
<span class="loding" style="display: none;"><span class="loding_txt">Loading</span></span>
</div>
<?php } ?>
<?php
}
}
?>
Well, isn’t it easy? Please feel free to share your thoughts and questions in the comment section below.
Happy Coding !!!
0 Comment(s)