This blog illustrate how to create a partial view in Asp.net MVC by using below steps:
-
Create a model class for partial view
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace PartialViewSampleAppliction.Models
{
public partial class PartialView
{
public string StudName { get; set; }
public int StudAge { get; set; }
}
public partial class PartialView
{
public List<PartialView> partialViewList { get; set; }
}
}
2. Create a Action method in Controller
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using PartialViewSampleAppliction.Models;
namespace PartialViewSampleAppliction.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
return View(new PartialView() { partialViewList = Details() });
}
private List<PartialView> Details()
{
List<PartialView> modelList = new List<PartialView>();
modelList.Add(new PartialView()
{
StudName = "Asha",
StudAge = 18,
});
modelList.Add(new PartialView()
{
StudName = "Amit",
StudAge = 20,
});
modelList.Add(new PartialView()
{
StudName = "Rashmi",
StudAge = 28,
});
return modelList;
}
}
}
3. To add a Partial View, Right click on Shared Folder inside 'Views' folder and click the 'Add' option in context menu then select the 'View... option'.
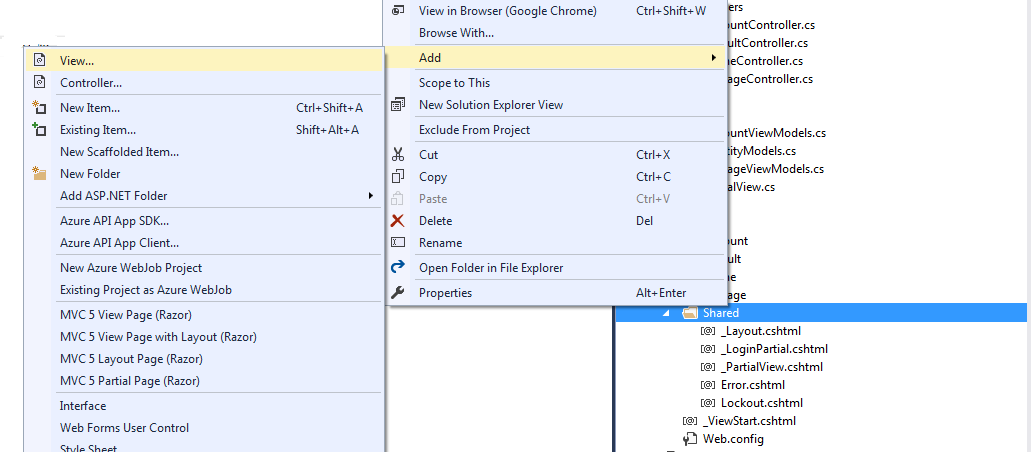
4. After selecting the 'View... option', we get a Add view window, here we need to provide a name of the partial view and check on the checkbox 'Create as a partial view' then click on 'Add' button.
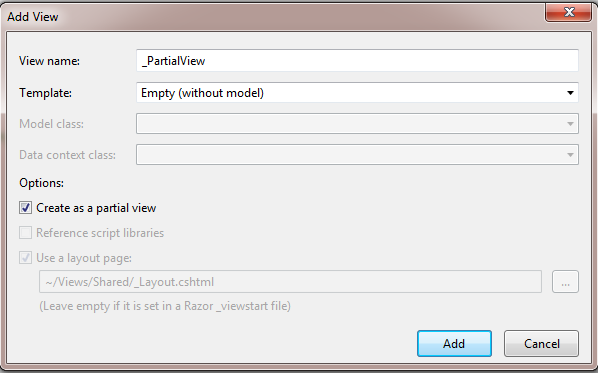
5. Add a below code in _PartialView.cshtml
@model IEnumerable<PartialViewSampleAppliction.Models.PartialView>
@if (Model != null)
{
<div class="grid">
<table cellspacing="0" width="80%">
<thead>
<tr>
<th>
Name
</th>
<th>
Age
</th>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<td align="left">
@item.StudName
</td>
<td align="left">
@item.StudAge
</td>
</tr>
}
</tbody>
</table>
</div>
}
6. Create a Index View which render a partial view(_PartialView.cshtml).
Add the below code in our Index View:
@model PartialViewSampleAppliction.Models.PartialView
@{
ViewBag.Title = "Index Page";
}
<p>
<div>
@Html.Partial("_PartialView", Model.partialViewList)
</div>
</p>
7. Run our project and get the below output.
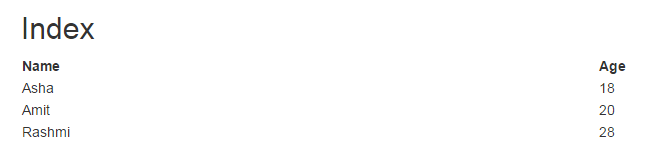
Conclusion:
When we run our project in web browser we get the list Students which is display by using a partial view.
0 Comment(s)