Logging is an act of keeping the record of all data inputs, processes, data outputs, and final results in a program. It is one of the most important parts of application maintenance. Logging helps in easy and quick identification of the bug in the code, thereby making the code more traceable.
In this blog, I will discuss, ways to centralize the logging process across the web applications using ELMAH (Error Logging Modules And Handlers).
However, before that let us have a look at ways to log exceptions or messages within a web application.
Creating a logging process within a web application is a very simple task, make a Logger class (either static or singleton as multiple instances are not required) with the following two methods i.e. LogInformation() & LogException.
public static class Logger
{
private const string logFilePath = "Path to the Log File......";
private const string InformationStart = "\n\n*******************Information Begin******************";
private const string InformationEnd = "*******************Information End******************\n\n";
private const string ExceptionStart = "\n\n*******************Exception Begin******************";
private const string ExceptionEnd = "***************************Exception End************************\n\n";
public static void LogInformation(string information)
{
using (System.IO.StreamWriter file = new System.IO.StreamWriter(HttpContext.Current.Server.MapPath(logFilePath), true))
{
file.WriteLine(InformationStart);
file.WriteLine(String.Format("{0} as on {1} :", "Information Log", String.Format("{0:f}", System.DateTime.Now)));
file.WriteLine(information);
file.WriteLine(InformationEnd);
}
}
public static void LogException(Exception exception)
{
StringBuilder exceptionDetails = new StringBuilder();
if (exception != null)
{
exceptionDetails.AppendLine(
string.Format(CultureInfo.CurrentCulture, "Exception Type:{0}", exception.GetType().Name));
exceptionDetails.AppendLine(
string.Format(CultureInfo.CurrentCulture, "Source:{0}", exception.Source));
exceptionDetails.AppendLine(
string.Format(CultureInfo.CurrentCulture, "Message:{0}", exception.Message));
exceptionDetails.AppendLine(
string.Format(CultureInfo.CurrentCulture, "Stack Tarce:{0}", exception.StackTrace));
using (System.IO.StreamWriter file = new System.IO.StreamWriter(HttpContext.Current.Server.MapPath(logFilePath), true))
{
file.WriteLine(ExceptionStart);
file.WriteLine(String.Format("{0} as on {1} :", "Exception Log", String.Format("{0:f}", System.DateTime.Now)));
file.WriteLine(exceptionDetails.ToString());
file.WriteLine(ExceptionEnd);
}
}
}
}
As the name itself signifies, LogInformation() can be used to log some information such as response, data input, data output, etc. whereas LogException() can be used to log all the exceptions that may occur while execution of the code. The above class also has a property 'logFilePath ', this property holds the path of the file where we are going to write all the logs of the application.
After creating this class, we can log any information or exception from anywhere throughout the application into a single LogFile whose path is defined in the logFilePath property of the Logger Class. Take the reference of this class and write Logger.LogInformation("Message to log") for logging any information and Logger.LogException("Exception to log") for logging any exception.
Note: Some of the most commonly used tools in .Net for logging is Log4Net,NLog,etc, the dll can be downloaded from Nuget.
Ideally, any process running on a system generates logs in some form or another. These logs are written to the files on the local disks (as we have seen above). When our system grows to a number of hosts, it gets nearly impossible to manage logs. An ideal solution for this situation is to establish a centralized logging solution so that multiple logs can be aggregated in a central location.
Setting up a centralized Logging across the web applications using ELMAH tool
ELMAH is an error logging open source library that can easily be downloaded from Nuget. It has various features such as error filtering and the capacity to view the error log from a web page which can also be downloaded as the comma-delimited file. It also provides an optional feature of mail on exception (i.e we get a mail on every exception logging). It is so simple to use that it can also be integrated into an existing website without making any change to the existing code. It also provides the detailed information of each exception that it logs, including the snapshot of “yellow page of death” which we usually see in the case of exception occurred.
ELMAH allows us to log exceptions using different formats such as Sql Server, SqLite, XML,etc. We will be using SqLite for logging as it provides an amazing database of the logs that can be accessed through any third party tool if required, and also helps in keeping the file size down which is not possible in while using XML files. The reason for not using Sql Server is that if any issue occurs with the server will result into not logging the exception
Follow these steps:
Step 1: Create an ASP.Net MVC application & Install ELMAH and System.Data.SQLite from nuget, this will create all the references and will also set up the web.config file. All we have to do is to make little modifications in the web.config file.
Step 2: Add the following line inside the <connectionstring> </connectionstring> tag
<add name="Elmah" connectionString="data source=~/Logs/ErrorLog.db" />
Step 3: Create a Log folder where the 'ErrorLog.db' file will be created. Now we are ready to go.
Step 4: Create a controller say SampleController write a code to create an exception for example:
public ActionResult GenerateError()
{
throw new Exception("Generate Error testing.");
return View();
}
Step 5: Run the application and call the above controller by writing the following command in your address bar http://YOUR_LOCAL_URL/Sample/GenerateError. (This Action Method will create an exception & that exception will be logged in the ErrorLog.db file.)
Step 6: Anyone who wants to view the exceptions can write http://YOUR_LOCAL_URL/elmah.axd in the address bar. This will give the complete list of all the exceptions occurred in the application at a centralized location with details option corresponding to each exception.
Example:
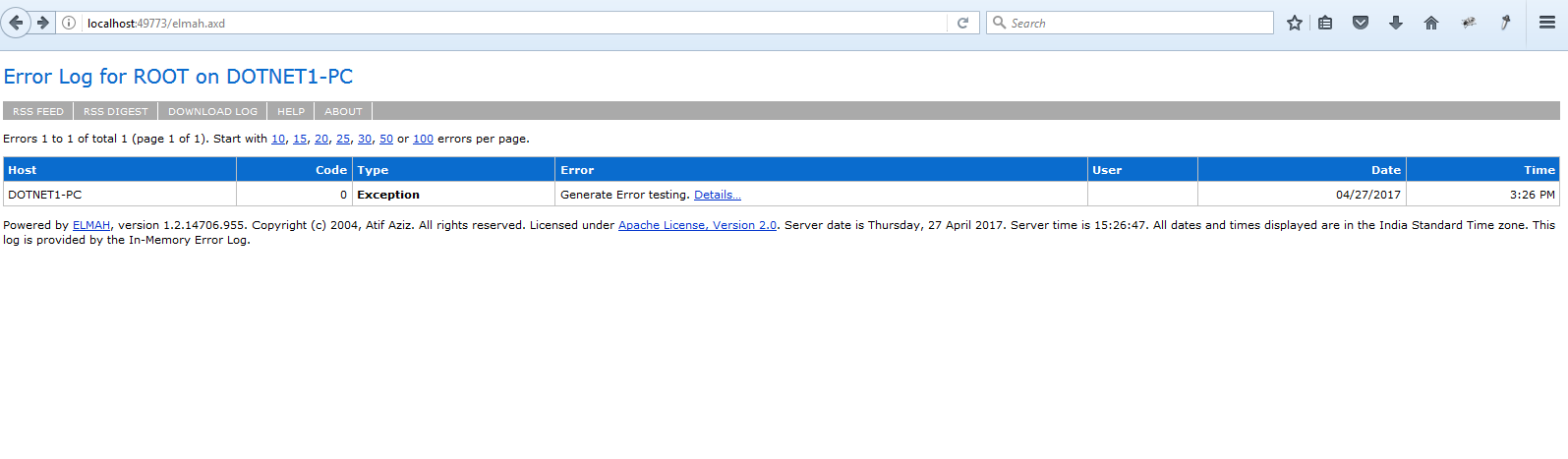
Happy Coding..!
Hope you like the write-up? Share your thoughts and comments in the comment section below.
0 Comment(s)