XSLT
XSL stands for eXtensible Stylesheet Language used for XML styling. XSLT in turn stands for XSL transformations i.e transformation of XML documents into different specific/design formats like XML transformation into Html.
Steps for transforming xml data as HTML using XSLT in ASP.NET MVC
Create an XML file "StudentInfo.xml". This file contains student information, xml content is as follows :-
<?xml version="1.0" encoding="utf-8" ?>
<StudentsInformation>
<Student Name="Himani" enrollmentdate="4/30/2005"></Student>
<Student Name="Deepak" enrollmentdate="3/4/2006"></Student>
<Student Name="Jyoti" enrollmentdate="1/20/2011"></Student>
<Student Name="Deepti" enrollmentdate="1/20/2001" ></Student>
</StudentsInformation>
Create an XSLT file "StudentInfo.xslt" and design it.
<?xml version="1.0" encoding="utf-8"?>
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform" xmlns:msxsl="urn:schemas-microsoft-com:xslt" exclude-result-prefixes="msxsl">
<xsl:template match="StudentsInformation">
<table cellpadding="0" cellspacing="0" border="1">
<tr style="text-align: center;color: blue;">
<th>Student Name</th>
<th>Enrollment Date</th>
</tr>
<xsl:for-each select="Student">
<tr style="text-align: center;color:red">
<td>
<xsl:value-of select="@Name"></xsl:value-of>
</td>
<td>
<xsl:value-of select="@enrollmentdate"></xsl:value-of>
</td>
</tr>
</xsl:for-each>
</table>
</xsl:template>
</xsl:stylesheet>
After XML and XSLT files are ready, XML data needs to be loaded and transform for displaying xml content.
Create a TransformationModel
namespace XMLParsingExample.Models
{
public class TransformationModel
{
public string XMLFilename { get; set; }
public string StylesheetFilename { get; set; }
}
}
Create HomeController with Index() action which is retrieving data from XML file.
public ActionResult Index()
{
string xmlFile = System.IO.File.ReadAllText(Server.MapPath("~/Data/StudentInfo.xml")); //Reading data from xml file
string xsltFile = Server.MapPath("~/Data/StudentInfo.xslt"); //path of xslt file
TransformationModel model = new TransformationModel();
model.XMLFilename = xmlFile;
model.StylesheetFilename = xsltFile;
return View(model);
}
Once content of xml file is retrieved, in order to view this xml content as Html a custom Html helper needs to be created. Custom Helper can be created in two ways :-
- Using HtmlHelper class extension method
A CustomHTMLHelper class with a static method RenderXml is created. RenderXml is an extension method which receives three parameters first parameter is the object of HtmlHelper class, second parameter is content of xml file and third parameter is path of xslt file and this helper returns a htmlString.
using System.IO;
using System.Web;
using System.Web.Mvc;
using System.Xml;
using System.Xml.Xsl;
namespace XMLParsingExample
{
public static class CustomHTMLHelper
{
public static HtmlString RenderXml(this HtmlHelper helper, string xml, string xsltPath)
{
XsltArgumentList args = new XsltArgumentList();
XslCompiledTransform tranformObj = new XslCompiledTransform(); // XslCompiledTransform object is created to load and compile XSLT file
tranformObj.Load(xsltPath);
XmlReaderSettings xmlSettings = new XmlReaderSettings(); // XMLReaderSetting object is created for assigning DtdProcessing, Validation type
xmlSettings.DtdProcessing = DtdProcessing.Parse;
xmlSettings.ValidationType = ValidationType.DTD;
// Create XMLReader object to Transform xml value with XSLT setting
using (XmlReader reader = XmlReader.Create(new StringReader(xml), xmlSettings))
{
StringWriter writer = new StringWriter();
tranformObj.Transform(reader, args, writer);
HtmlString htmlString = new HtmlString(writer.ToString()); //HTML string from StringWriter is generated
return htmlString;
}
}
}
}
Above CustomHelper can be called in view (Index.cshtml) in following way :-
@model XMLParsingExample.Models.TransformationModel
<div data-role="content">
@Html.RenderXml(Model.XMLFilename, Model.StylesheetFilename)
</div>
A static class CustomHTMLHelper with static method RenderXml is created. Static method only receives content of xml file and path of xslt file returning a htmlString.
using System.IO;
using System.Web;
using System.Xml;
using System.Xml.Xsl;
namespace XMLParsingExample
{
public static class CustomHTMLHelper
{
public static HtmlString RenderXml(string xml, string xsltPath)
{
XsltArgumentList args = new XsltArgumentList();
// Create XslCompiledTransform object to loads and compile XSLT file.
XslCompiledTransform tranformObj = new XslCompiledTransform();
tranformObj.Load(xsltPath);
// Create XMLReaderSetting object to assign DtdProcessing, Validation type
XmlReaderSettings xmlSettings = new XmlReaderSettings();
xmlSettings.DtdProcessing = DtdProcessing.Parse;
xmlSettings.ValidationType = ValidationType.DTD;
// Create XMLReader object to Transform xml value with XSLT setting
using (XmlReader reader = XmlReader.Create(new StringReader(xml), xmlSettings))
{
StringWriter writer = new StringWriter();
tranformObj.Transform(reader, args, writer);
// Generate HTML string from StringWriter
HtmlString htmlString = new HtmlString(writer.ToString());
return htmlString;
}
}
}
}
The logic is similar to extension Helper class but is called differently.
@model XMLParsingExample.Models.TransformationModel
<div data-role="content">
@CustomHTMLHelper.RenderXml(Model.XMLFilename, Model.StylesheetFilename)
</div>
After running the application, in both cases xml content will be displayed in html format.
Output :-
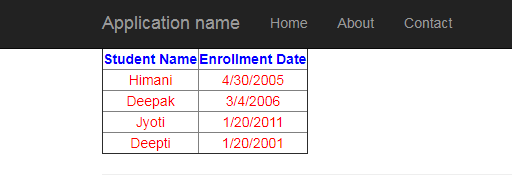
0 Comment(s)