We can easily create a simple table view to show the data in tabular format but if we need to show UICollectionView inside the UITableViewCell then it become a challenging task for the beginners. So here is an easy tutorial, to get the concept of how to show a UICollectionView in a UITableViewCell using swift language.
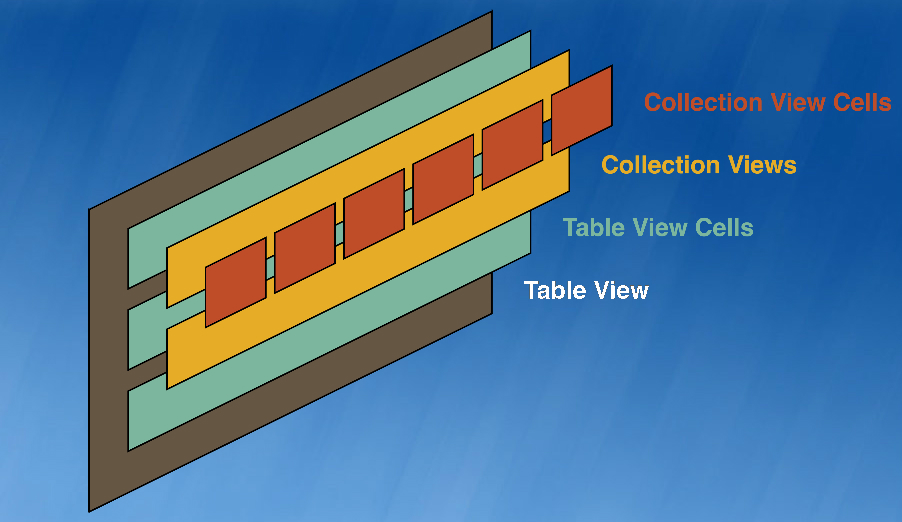
We will start with the user interface part, we will take a UITableView with UITableViewCell and inside UITableViewCell put UICollectionView, as given in the screenshot below:-
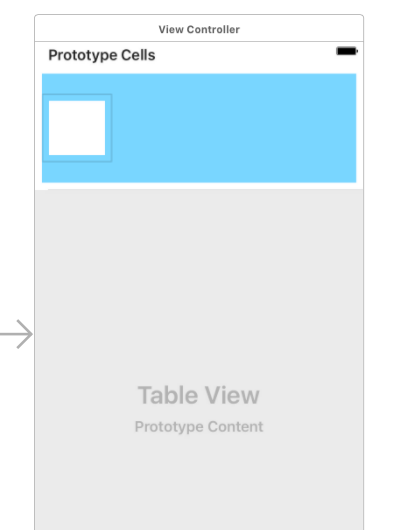
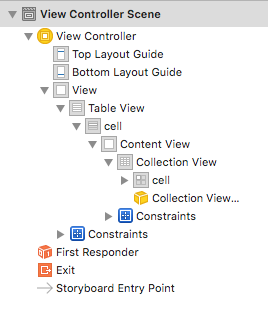
Now we will move towards the coding part here before proceeding set datasource and delegate of UITableView, if these two are not connected then you will not be able to get the appropriate result.
After setting datasource and delegate through main.storyboard go to the ViewController file where further methods are written. In this file, we will inherit the class with UITableViewDelegate and UITableViewDataSource so that we will be able to access their methods.
Here we will define how many rows we required in UITableView with method numberOfRowsInSection and return cell with the cellForRowAt method. Assign new TableViewCell class to the UITableViewCell and provide identifier named cell.
So in this file, we are only providing the number of rows and returning the cell.
//
// ViewController.swift
// CollectionViewInsideTableViewCell
//
// Created by elcaptain1 on 9/19/17.
// Copyright 2017 Tanuja. All rights reserved.
//
import UIKit
class ViewController: UIViewController,UITableViewDelegate,UITableViewDataSource {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return 10
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "cell", for: indexPath) as! TableViewCell
return cell
}
}
Outlet of the view inside the UICollectionViewCell is taken in this file.
//
// CollectionViewCell.swift
// CollectionViewInsideTableViewCell
//
// Created by elcaptain1 on 9/19/17.
// Copyright 2017 Tanuja. All rights reserved.
//
import UIKit
class CollectionViewCell: UICollectionViewCell {
@IBOutlet weak var view: UIView!
}
In this file, we will inherit the class with UICollectionViewDelegate and UICollectionViewDataSource also as we need to show UICollectionView inside UITableViewCell.
Provide new class CollectionViewCell to the cell of collection view and identifier with name cell. Here also we will provide two methods to tell numberOfItemsInSection and cellForItemAt.
We have taken an array of UIColor as we have taken a view inside collection view cell and we will set all those colors in the view.
//
// TableViewCell.swift
// CollectionViewInsideTableViewCell
//
// Created by elcaptain1 on 9/19/17.
// Copyright 2017 Tanuja. All rights reserved.
//
import UIKit
class TableViewCell: UITableViewCell,UICollectionViewDelegate,UICollectionViewDataSource {
var arrayOfColor = [UIColor]()
@IBOutlet weak var collectionView: UICollectionView!
override func awakeFromNib() {
super.awakeFromNib()
collectionView.dataSource = self as UICollectionViewDataSource
collectionView.delegate = self as UICollectionViewDelegate
arrayOfColor = [UIColor.red,UIColor.blue,UIColor.gray,UIColor.brown,UIColor.green,UIColor.cyan,UIColor.darkGray,UIColor.darkText]
// Initialization code
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
// Configure the view for the selected state
}
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return arrayOfColor.count
}
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "cell", for: indexPath) as! CollectionViewCell
cell.view.backgroundColor = arrayOfColor[indexPath.row]
return cell
}
}
I hope this tutorial will help you out in showing UICollectionView inside UITableViewCell. Feel Free to ask any question regarding this tutorial. You can also download the sample code from the file attached below
0 Comment(s)