If you are developing a web application or any website, you should have to take a stem to store your user's admin password securely in your database.
Secure way means that the way to save your password in your database in an encrypted way by which it should not be readable by any other person who can access your web application database. If the password to the database is compromised then the whole database can be compromised and can be retrieved by anyone.
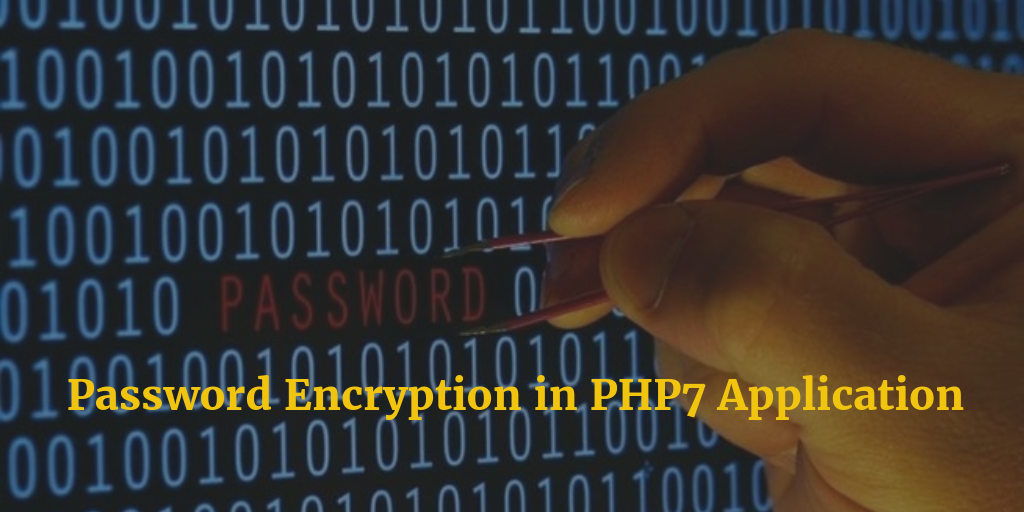
We can put that security to encrypt all passwords in our database using Message Digest Algorithm 5 that is known as MD5.MD5 encryption is a one-way hashing algorithm that is used to encrypt and text string. In the MD5 algorithm, it is impossible to revert back any encrypted data into the plain-text input. This ensures that the passwords stored on the server cannot be readable to anyone. But we have to make sure that all the users should have strong passwords for logging in.
In the MD5 algorithm, it is impossible to revert back any encrypted data into the plain-text input. This ensures that the passwords stored on the server can't be readable by anyone. But we have to make sure that all the users should have strong passwords for logging in, it's because MD5 has its weakness, if the user password is weak it can be retrieved by some experts easily. MD5 is not too much secure but we use it because it's easy and fast, and it can be powerful if salted.
So in this tutorial, We will learn Password Encryption in PHP7, below are the simple steps:
- For adding password encryption functionality in PHP, first, you need to create a new account and this can be done by using below code=>
<!-- code for creating database connection-->
<?php
define("DB_SERVER", "localhost");
define("DB_USER", "your_name");
define("DB_PASS", "your_pass");
define("DB_NAME", "your_db");
define("TBL_USERS", "users_table_name");
$connection = mysql_connect(DB_SERVER, DB_USER, DB_PASS) or die(mysql_error());
mysql_select_db(DB_NAME, $connection) or die(mysql_error());
// database connection code ends
// function for creating new user
function addNewUser($username, $password){
global $connection;
$password = md5($password); // password will be encrypted automatically.
$q = "INSERT INTO ".TBL_USERS." VALUES ('$username', '$password')";
return mysql_query($q, $connection);
}
?>
- After then you can create a form for registration and can send a request to this function by which new account can be created.
- Once the user makes an account, we should write a code for validating a given username/password.
<?php
function checkUserPass($username, $password){
global $connection;
$username = str_replace("'","''",$username) // replacing unusual spaces
$password = md5($password);
// Verify that user is in database
$q = "SELECT password FROM ".TBL_USERS." WHERE username = '$username'"; //checking if username exists in database
$result = mysql_query($q, $connection);
if(!$result || (mysql_numrows($result) < 1)){
return 1; //Indicates username failure
}
// Retrieve password from result
$dbarray = mysql_fetch_array($result);
// Validate that password is correct
if($password == $dbarray['password']){
return 0; //Success! Username and password confirmed
}
else{
return 1; //Indicates password failure
}
}
?>
- And if you already have a user in your database then we have to encrypt the password. To encrypt, we need to write an encrypt.php script with the following code.
<?php
define("DB_SERVER", "localhost");
define("DB_USER", "your_name");
define("DB_PASS", "your_pass");
define("DB_NAME", "your_db");
define("TBL_USERS", "users_table_name");
define("FLD_USER", "username_field_name");
define("FLD_PASS", "password_field_name");
set_magic_quotes_runtime(0);
$connection = mysql_connect(DB_SERVER, DB_USER, DB_PASS) or die(mysql_error());
mysql_select_db(DB_NAME, $connection) or die(mysql_error());
$q = "SELECT ".FLD_PASS.",".FLD_USER." FROM ".TBL_USERS."";
$result = mysql_query($q, $connection);
$total=0;
$enc=0;
$doencrypt=false;
if (@$_REQUEST["do"]=="encrypt")
$doencrypt=true;
while($data = mysql_fetch_array($result))
{
if ($doencrypt)
{
$total++;
if (!encrypted($data[0]))
{
$q="UPDATE ".TBL_USERS." SET ".FLD_PASS."='".md5($data[0])."' where ".FLD_USER."='".
str_replace("'","''",$data[1])."'";
mysql_query($q, $connection);
}
$enc++;
}
else
{
$total++;
if (encrypted($data[0]))
$enc++;
}
}
function encrypted($str)
{
if (strlen($str)!=32)
return false;
for($i=0;$i<32;$i++)
if ((ord($str[$i])<ord('0') || ord($str[$i])>ord('9')) && (ord($str[$i])<ord('a') || ord($str[$i])>ord('f')))
return false;
return true;
}
?>
<html>
<head><title>passwords</title></head>
<body>
<?php echo $total; ?><br>
<?php if($enc==$total && $total>0) { ?>
All passwords are encrypted.
<?php } else if($total>0) { ?>
Unencrypted - <?php echo $total-$enc; ?><br><br>
<?php echo $total-$enc; ?> passwords.<br>
<input type=button value="GO" onclick="window.location='encrypt.php?do=encrypt';">
<?php } ?>
</body>
</html>
1 Comment(s)