AngularJS is an open source free and most popular JavaScript client-side framework which provides a great power to built HTML and JavaScript based web application.
This tutorial is in continuation with my previous tutorials, regarding AngularJs and if you are beginner then first go to the intoductory tutorials mentioned below:
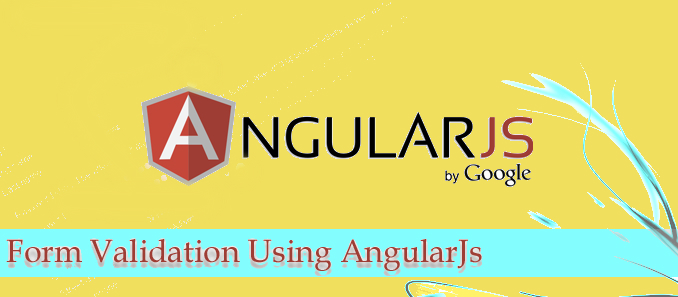
This tutorial is all about Simple AngularJs Validation Form. Here, Validation is basically monitoring the state of form and input fields and then later provides the current state about the input and form to the user and this all is done by Angularjs Validation.
In this blog I am sharing a simple example in which user will register himself and once the user will be registered he/she can check their details.
Different type of Angularjs Validation features is used in this example.
Validation features in input tags:
-
$pristine
: This property is used to ensure that the input field has not been modified.
-
$dirty
: This property is used to ensure that the input field has been modified.
-
$invalid :
This property is used to ensure that the input field content is not valid.
-
$valid
: This property is used to ensure that the input field content is valid.
-
$untouched
:This property is used to ensure that the input field has not been touched.
-
$touched
: This property is used to ensure that the input field field has been touched.
Validation features in Form:
-
$pristine :
This property is used to ensure that none of the form fields have been modified.
-
$dirty
:
This property is used to ensure that One or more form fields have been modified.
-
$invalid
: This property is used to ensure that the form content is not valid.
-
$valid
: This property is used to ensure that the form content is valid.
-
$submitted :
This property is used to ensure that the form is submitted successfully.
Please go through the example below to understand how we can use angularjs to register user.
In this example user can change their information by using edit and delete feature even after getting registered once.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Angularjs Form</title>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="./css/bootstrap.min.css">
<script src="./js/angular.js"></script>
<script type="text/javascript" src="./js/app.js"></script>
<link rel="stylesheet" type="text/css" href="./css/style.css">
</head>
<body ng-app="myApp">
<div class="container clr" ng-controller="AppController as ctrl">
<h1>Registration Form</h1>
<div class="panel panel-default subcontainer" >
<div class="panel-heading"><span class="lead">Register YourSelf</span></div>
<div class="formcontainer">
<form ng-submit="ctrl.submit()" name="myForm" >
<input type="hidden" ng-model="ctrl.user.id" />
<div class="row">
<div class="col-md-12">
<label class="col-md-2" for="uname">Name</label>
<div class="col-md-10">
<input type="text" name="username" ng-model="ctrl.user.username" id="uname" class="nameField form-control input-sm" placeholder="Enter your name" required ng-minlength="3"/>
<div ng-show="myForm.$dirty">
<span ng-show="myForm.username.$error.required">This is a required field</span>
<span ng-show="myForm.username.$error.minlength">Minimum length required is 3</span>
<span ng-show="myForm.username.$invalid">This field is invalid </span>
</div>
</div>
</div>
</div>
<div class="row">
<div class="col-md-12">
<label class="col-md-2" for="password">Password</label>
<div class="col-md-10">
<input type="password" ng-model="ctrl.user.password" id="password" class="passwordField form-control input-sm" placeholder="Enter your password." ng-minlength="5" name="password"
required/>
<div ng-show="myForm.$dirty">
<span ng-show="myForm.password.$error.required">This is a required field</span>
<span ng-show="myForm.password.$error.minlength">Minimum length required is 5</span>
<span ng-show="myForm.password.$invalid">This field is invalid </span>
</div>
</div>
</div>
</div>
<div class="row">
<div class="col-md-12">
<label class="col-md-2" for="email">Email</label>
<div class="col-md-10">
<input type="email" name="email" ng-model="ctrl.user.email" id="email" class="emailField form-control input-sm" placeholder="Enter your Email" required/>
<div ng-show="myForm.$dirty">
<span ng-show="myForm.email.$error.required">This is a required field</span>
<span ng-show="myForm.email.$invalid">This field is invalid </span>
</div>
</div>
</div>
</div>
<div class="row submitContainer">
<div class="col-md-6">
<input type="submit" ng-value="Add" class="btn btn-primary btn-sm" ng-disabled="myForm.$invalid">
</div>
<div class="col-md-6"><button type="button" ng-click="ctrl.reset()" class="btn btn-warning btn-sm" ng-disabled="myForm.$pristine">Reset</button></div>
</div>
</form>
</div>
</div>
<div class="panel panel-default subcontainer">
<!-- Default panel contents -->
<div class="panel-heading"><span class="lead">Check Your Details</span></div>
<div class="tablecontainer">
<table class="table table-hover">
<thead>
<tr>
<th>ID.</th>
<th>Name</th>
<th>Email</th>
<th>Password</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="u in ctrl.users">
<td><span ng-bind="u.id"></span></td>
<td><span ng-bind="u.username"></span></td>
<td><span ng-bind="u.email"></span></td>
<td><span ng-bind="u.password"></span></td>
<td> <button type="button" ng-click="ctrl.edit(u.id)" class="btn btn-success custom-width">Edit</button> <button type="button" ng-click="ctrl.delete(u.id)" class="btn btn-danger custom-width">Delete</button></td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
</div>
</body>
</html>
CSS:
/* background color of input field :username to show Error type */
.nameField.ng-valid {background-color: lightgreen;}
.nameField.ng-dirty.ng-invalid-required {background-color: red;}
.nameField.ng-dirty.ng-invalid-minlength {background-color: yellow;}
/* background color of input field email to show Error type */
.emailField.ng-valid {background-color: lightgreen;}
.emailField.ng-dirty.ng-invalid-required {background-color: red;}
.emailField.ng-dirty.ng-invalid-email {background-color: yellow;}
/* background color of input field password to show Error type */
.passwordField.ng-valid {background-color: lightgreen;}
.passwordField.ng-dirty.ng-invalid-required {background-color: red;}
.passwordField.ng-dirty.ng-invalid-email {background-color: yellow;}
/* customised css*/
body{background-color:rgba(0,0,0,0.5);}
h1 {text-align: center;padding: 20px;margin: 0 auto;color:#fff;text-shadow: 3px 0px 2px #000;}
.subcontainer{padding:0;}
form{margin:0 auto;}
.row{margin:10px auto;}
.formcontainer {margin: 20px auto 0;}
.submitContainer {
text-align: center;
border-top: 1px solid #ddd;
margin: 0 auto;
padding: 15px 0;
background-color: #f5f5f5;
}
Angularjs:
angular.module('myApp', [])
.controller('AppController', ['$scope', function($scope) {
this.user={id:null,username:'',address:'',email:''};
this.id = null;
$scope.Add = "Add";
this.users = [];
this.submit = function() {
if(this.user.id === null){
this.user.id = this.id++;
console.log('Saving New User', this.user);
this.users.push(this.user);//Or send to server, we will do it in when handling services
}else{
for(var i = 0; i < this.users.length; i++){
if(this.users[i].id === this.user.id) {
this.users[i] = this.user;
break;
}
}
console.log('User updated with id ', this.user.id);
}
this.reset();
};
this.edit = function(id){
console.log('id to be edited', id);
for(var i = 0; i < this.users.length; i++){
if(this.users[i].id === id) {
this.user = angular.copy(this.users[i]);
$scope.Add = "Update";
break;
}
}
}
this.remove = function(id){
console.log('id to be deleted', id);
for(var i = 0; i < this.users.length; i++){
if(this.users[i].id === id) {
this.users.splice(i,1);
if(this.user.id === id){//It is shown in form, reset it.
this.reset();
}
break;
}
}
}
this.reset = function(){
this.user={id:null,username:'',address:'',email:''};
$scope.myForm.$setPristine(); //reset Form
}
}]);
How you liked the example of Angularjs Form Validation, please feel free to share your thoughts in comment section below.
0 Comment(s)