In this tutorial, we will see how to Fetch Particular Substring from a String in iOS Swift3 Program, but before moving on the mane case first of all let us see, What is String and Substring?
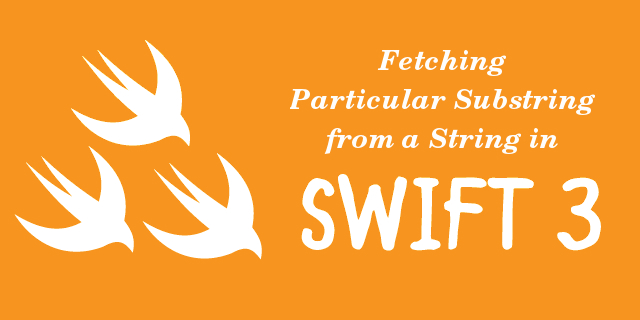
What is String
String can be defined as the collection of characters for example "Hi". The String can be used for a various purpose in a program and whole value of the string can be directly used in the program.
String can be declared in the given way below:
let sampleString = String() //declaration
sampleString = "Hello" // initialisation
What is Substring
Substring can be defined as any part of a particular string fetched according to the need. A substring of a string can find out in different ways but here is an example to find out a substring from a particular part of a string. One of the methods to identify substring is given below:-
let str = "Hello, playground"
print(str.substring(from: 7)) // playground
String and substring are interconnected as to find out substring we need a string and string is a collection of any number of substrings.
Code of extension file is given below:-
This extension file includes two methods in it and both have different functionality. Function removePrefix is used to remove the number of characters from the starting of a string and the other method removeSuffix is used to remove the number of characters from the end of a string.
//
// StringExtension.swift
// Sample1
//
// Created by elcaptain1 on 8/1/17.
// Copyright 2017 Tanuja. All rights reserved.
//
import Foundation
extension String {
func removePrefix(_ count: Int = 1) -> String {
return substring(from: index(startIndex, offsetBy: count))
}
func removeSuffix(_ count: Int = 1) -> String {
return substring(to: index(endIndex, offsetBy: -count))
}
}
Code to identify the particular substring from a string
So below is the code for the same let us assume a string str which includes data and firstly we will create an array of elements from the string which are separated by space. When an array is created then we can easily identify substrings by index. According to index substrings can be identified but now we need to remove some letters from the front and last from substring so we will create an extension file with two methods which are used to remove letters from start and end of a substring.
//
// ViewController.swift
// Sample1
//
// Created by elcaptain1 on 8/1/17.
// Copyright 2017 Tanuja. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let str = "<message type=\"chat\" to=\"chat123@ec2-34-206-125-80.compute-1.amazonaws.com\" from=\"newuser1@ec2-34-206-125-80.compute-1.amazonaws.com\" id=\"DD7D1322-4258-49DD-B1DD-E5F151B2E5C5\"><body>Hi</body></message"
let first = str.components(separatedBy: " ")
var toString = first[2]
toString = toString.removePrefix(4)
toString = toString.removeSuffix(1)
var fromString = first[3]
fromString = fromString.removePrefix(6)
fromString = fromString.removeSuffix(1)
print(substring first == \(toString)")
print("substring second == \(fromString)")
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
Output:-
substring first == chat123@ec2-34-206-125-80.compute-1.amazonaws.com
substring second == newuser1@ec2-34-206-125-80.compute-1.amazonaws.com
0 Comment(s)