Before moving on to the topic first of all you must be aware with Firebase, if not then please, do visit this my last tutorial on How to Register Realtime User Information at Firebase in iOS. It will clear you about how to get register in Firebase through the application and how to use Firebase.
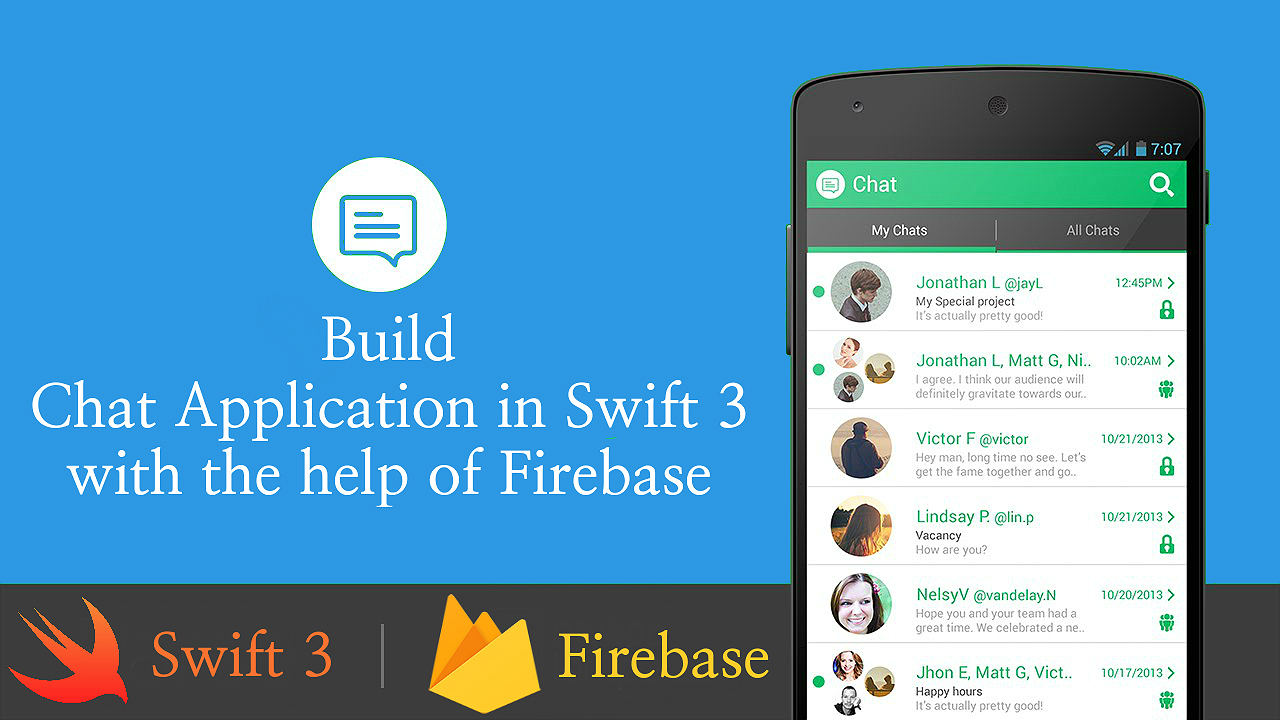
Now in this part, I will explain the about Login and chat, so we are going to build a chat application in a swift language with the help of Firebase and for that first of all, please do create a separate project for chat application to get more information of Firebase.
First of all, user interface of the chat application includes two controllers:-
1. TableViewController - This controller is used to represent the username registered in Firebase.
2. ChatViewController - Here we can see how to send a message in real time and how they are going to save in Firebase.
Screenshot of Main.storyboard is given below:-
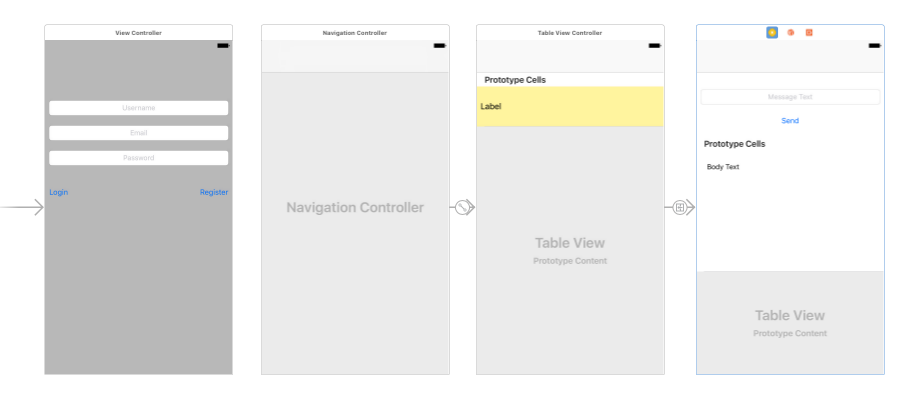
Now, coming towards the coding part one by one we will start the coding as first of all, for login we need to code as in the previous blog (goo.gl/3cTRJn) only registration of user information is done.
Below code includes the login functionality and if the user is logged in it will directly take the user to the home screen where we can see how many users are registered and on tap in any username we will move towards the next controller.
In ChatViewController we can send a message and those messages will be seen in the tableview given on the screen.
//
// TableViewController.swift
// ChatApplication
//
// Created by elcaptain1 on 9/22/17.
// Copyright 2017 Tanuja. All rights reserved.
//
import UIKit
import Firebase
import FirebaseAuth
import FirebaseCore
import FirebaseDatabase
import Foundation
var currentUserChatId = String()
struct User {
let userName:String!
let uId:String!
}
class TableViewController: UITableViewController {
var users = [User]()
override func viewDidLoad() {
super.viewDidLoad()
let uid = FIRAuth.auth()?.currentUser?.uid
let database = FIRDatabase.database().reference()
database.child("users").queryOrderedByKey().observe(.childAdded, with: {snapshot in
let username = (
snapshot.value as? NSDictionary
)?["username"] as? String ?? ""
let uid = (
snapshot.value as? NSDictionary
)?["uid"] as? String ?? ""
self.users.append(User(userName:username,uId:uid))
self.tableView.reloadData()
})
tableView.reloadData()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// MARK: - Table view data source
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
// #warning Incomplete implementation, return the number of rows
return users.count
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "cell", for: indexPath)
let usernameTextField = cell.viewWithTag(1) as! UILabel
usernameTextField.text = users[indexPath.row].userName
return cell
}
override func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
currentUserChatId = users[indexPath.row].uId
}
}
//
// ChatViewController.swift
// ChatApplication
//
// Created by elcaptain1 on 9/22/17.
// Copyright 2017 Tanuja. All rights reserved.
//
import UIKit
import Firebase
import FirebaseAuth
import FirebaseCore
import FirebaseDatabase
struct Post {
let bodyText:String!
}
class ChatViewController: UIViewController,UITableViewDelegate,UITableViewDataSource {
@IBOutlet weak var txtMessage: UITextField!
@IBOutlet weak var tableView: UITableView!
var posts = [Post]()
override func viewDidLoad() {
super.viewDidLoad()
tableView.delegate = self
tableView.dataSource = self
let database = FIRDatabase.database().reference()
database.child("posts").child(currentUserChatId).queryOrderedByKey().observe(.childAdded, with:
{
snapshot in
let bodyText = (
snapshot.value as? NSDictionary
)?["bodyText"] as? String ?? ""
self.posts.insert(Post(bodyText:bodyText), at: 0)
self.tableView.reloadData()
})
tableView.reloadData()
// Do any additional setup after loading the view.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return posts.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "cell", for: indexPath)
let postTextView = cell.viewWithTag(1) as! UITextView
postTextView.text = posts[indexPath.row].bodyText
return cell
}
@IBAction func btnSend(_ sender: AnyObject) {
if txtMessage.text != nil {
let uid = FIRAuth.auth()?.currentUser?.uid
let database = FIRDatabase.database().reference()
let bodyData :[String:Any] = ["uid":uid!,"bodyText":txtMessage.text!]
database.child("posts").child(currentUserChatId).childByAutoId().setValue(bodyData)
}
}
}
Output:-
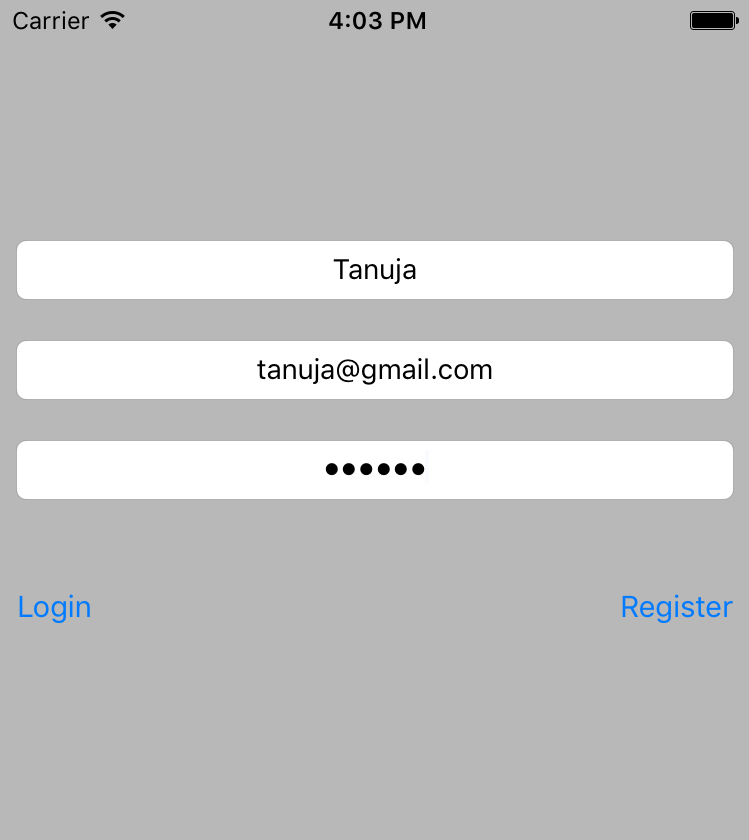
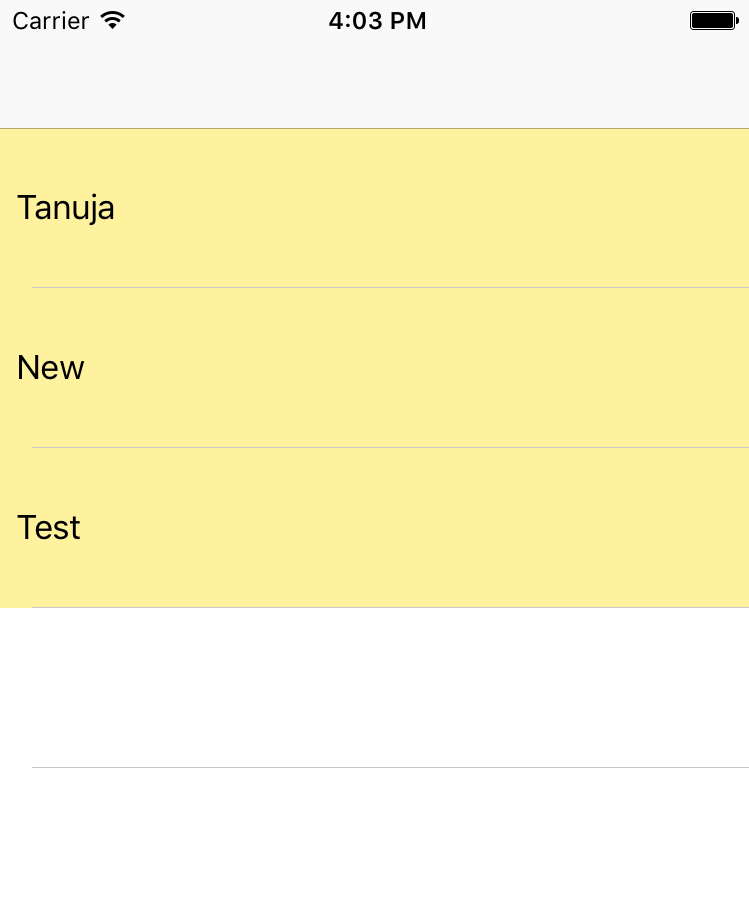
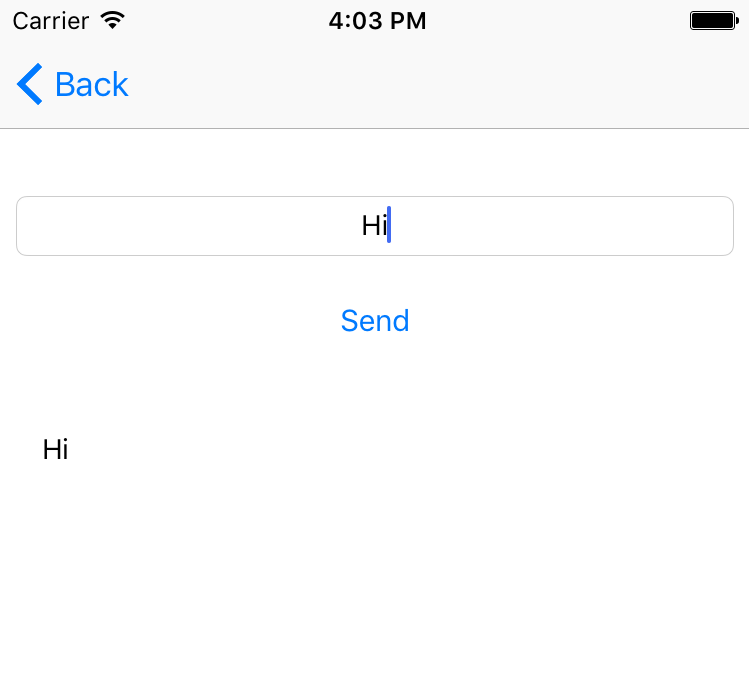
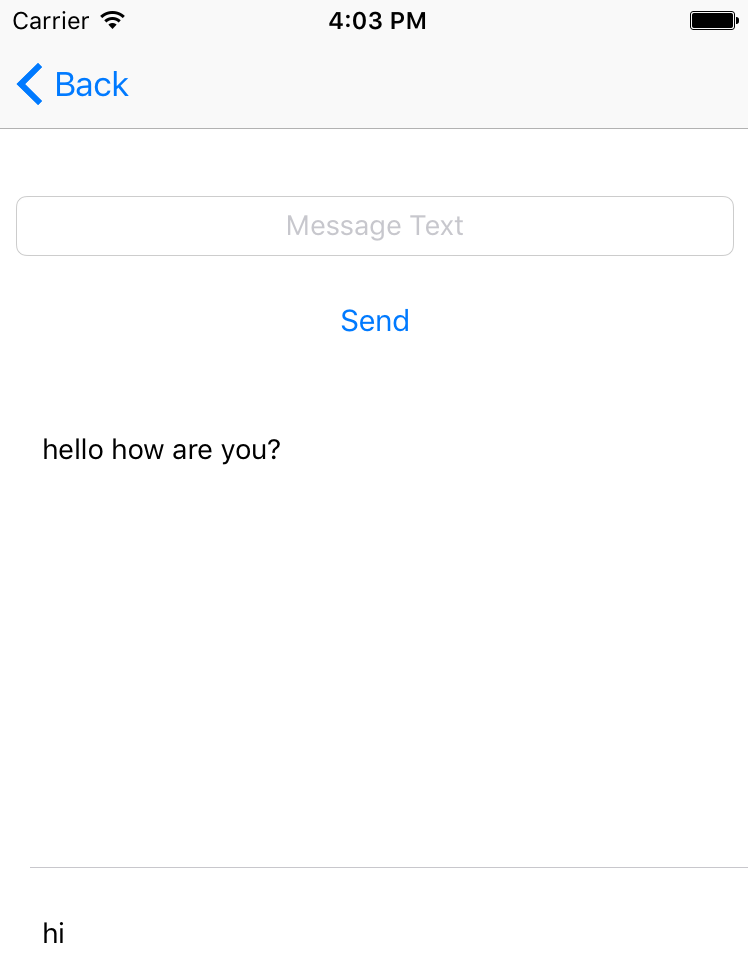
You can also download the sample code from link given below.
0 Comment(s)