Tic-tac-toe is a very well known game among young children and very often most of us like to play this game to remove stress and feel refreshed. This game is for two players and all about placing noughts and crosses or Xs and Os in any place in a 3x3 grid and if anyone places three noughts or crosses in diagonal, vertical or horizontal wins the game. Here is the way how this game can be created for iPhone users with the help of swift language.
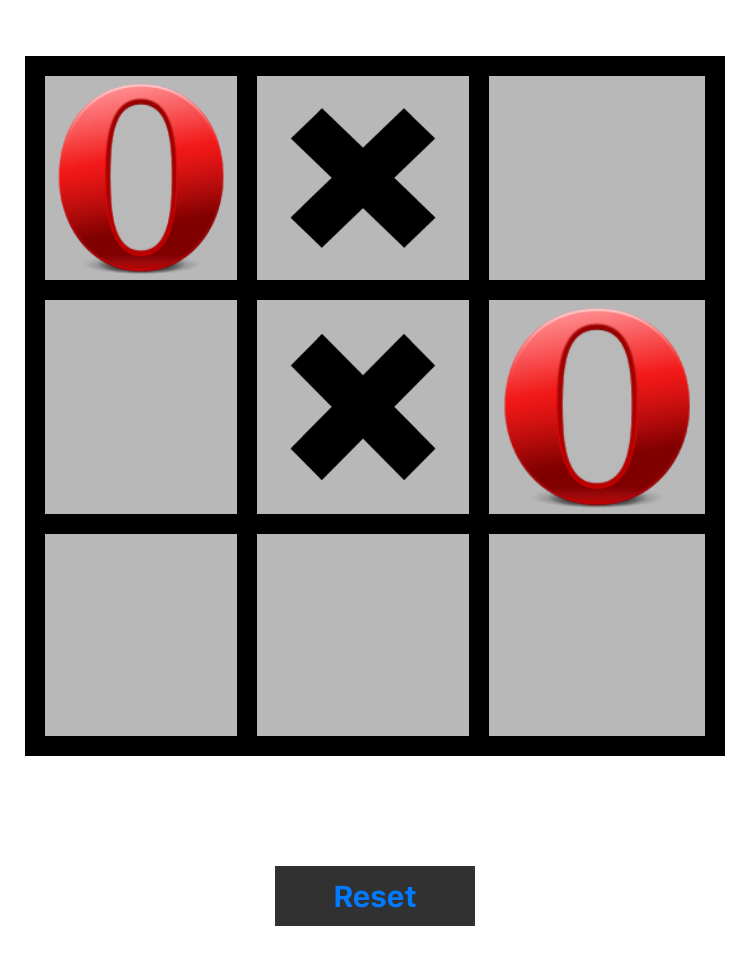
Only one view controller is needed for the functionality part of the game. In view controller four variables are used for different purpose:-
- The activePlayerOfGame variable is used to identify the active player so that next move will be done by other active players.
- The stateOfGame is an array is used to provide the states of the game when user start playing and as the number of fields fills state of the game will get changed.
- The possibleCombinationToWinMatch is an array which provides the possible number of combination for the user to be set as the winner.
- The isGameActive variable is a boolean value to identify game is active and if the game is active only then further processing will be done.
Button action of btnPlay is used for further processing like checking the condition to set image according to the player and who won the match either Xs or 0s.
Below are the code and screenshot of creating the user interface and functionality for the same:
//
// ViewController.swift
// TicTacToeGameApplication
//
// Created by elcaptain1 on 7/14/17.
// Copyright 2017 Tanuja. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
var activePlayerOfGame = 1 //cross
var stateOfGame = [0,0,0,0,0,0,0,0,0]
var possibleCombinationToWinMatch = [[0,1,2],[3,4,5],[6,7,8],[0,3,6],[1,4,7],[2,5,8],[0,4,8],[2,4,6]]
var isGameActive = true
@IBAction func btnPlay(_ sender: AnyObject) {
if(stateOfGame[sender.tag-1] == 0 && isGameActive == true)
{
stateOfGame[sender.tag-1] = activePlayerOfGame
if(activePlayerOfGame == 1)
{
sender.setImage(UIImage(named: "cross.png"), for: .normal)
activePlayerOfGame = 2
}
else{
sender.setImage(UIImage(named: "0.png"), for: .normal)
activePlayerOfGame = 1
}
}
for combination in possibleCombinationToWinMatch {
if stateOfGame[combination[0]] != 0 && stateOfGame[combination[0]] == stateOfGame[combination[1]] && stateOfGame[combination[1]] == stateOfGame[combination[2]]
{
isGameActive = false
if stateOfGame[combination[0]] == 1
{
let alert = UIAlertView(title: "TicTacToe", message: "Cross won the match", delegate: self, cancelButtonTitle: "Ok")
alert.show()
print("cross")
DispatchQueue.main.asyncAfter(deadline: .now() + 1.0, execute: {
self.playAgain()
})
}
else
{
let alert = UIAlertView(title: "TicTacToe", message: "Zero won the match", delegate: self, cancelButtonTitle: "Ok")
alert.show()
print("zero")
DispatchQueue.main.asyncAfter(deadline: .now() + 1.0, execute: {
self.playAgain()
})
}
}
}
isGameActive = false
for i in stateOfGame {
if i == 0
{
isGameActive = true
break
}
}
if(isGameActive == false)
{
let alert = UIAlertView(title: "TicTacToe", message: "Match draw", delegate: self, cancelButtonTitle: "Ok")
alert.show()
DispatchQueue.main.asyncAfter(deadline: .now() + 1.0, execute: {
self.playAgain()
})
print("zero")
}
print(sender.tag)
}
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func btnPlayAgain(_ sender: AnyObject) {
playAgain()
}
func playAgain() {
stateOfGame = [0,0,0,0,0,0,0,0,0]
isGameActive = true
for i in 1...9 {
let button = view.viewWithTag(i) as!UIButton
button.setImage(nil, for: .normal)
}
}
}
All the screenshot are below to make this concept more understandable.
First one includes two controllers to tell how to design the screens. The first screen only includes one label with the title of the game and one button which take you to the next screen where dashboard of the game is available and one button to reset the dashboard. The dashboard screen is designed with view and buttons as a grid of 3x3 is the requirement of the game.
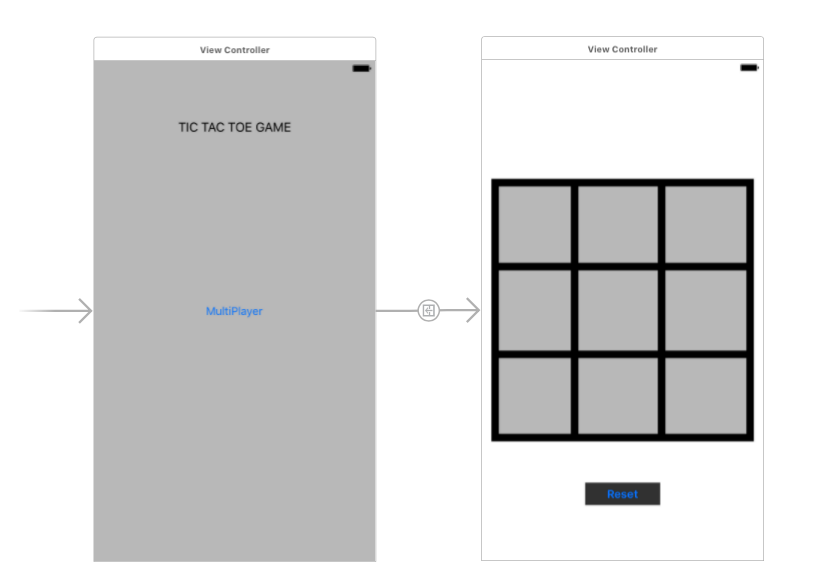
Other screens include the layout after execution of application followed by how the user will get to know who won the match.
2nd Screen
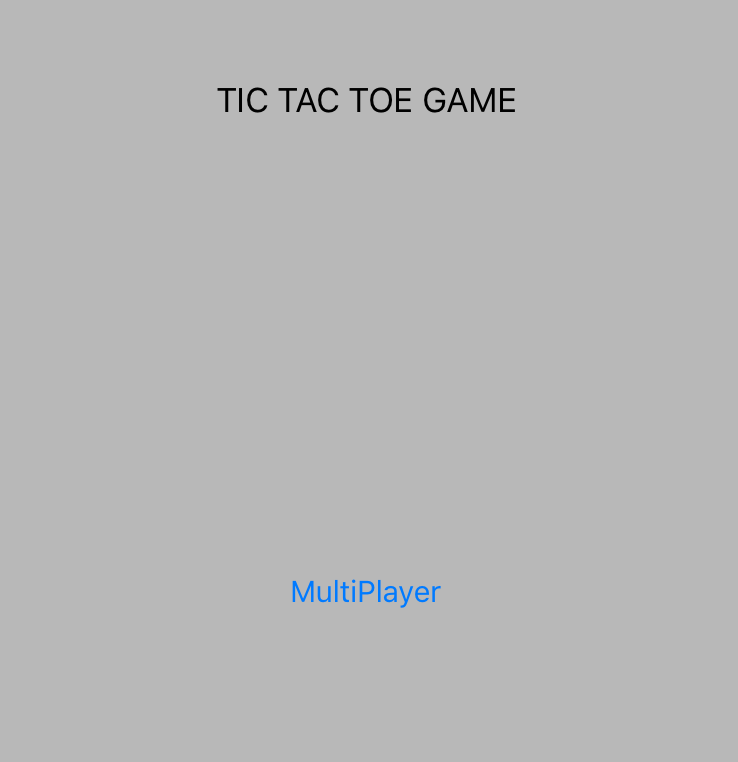
3rd Screen
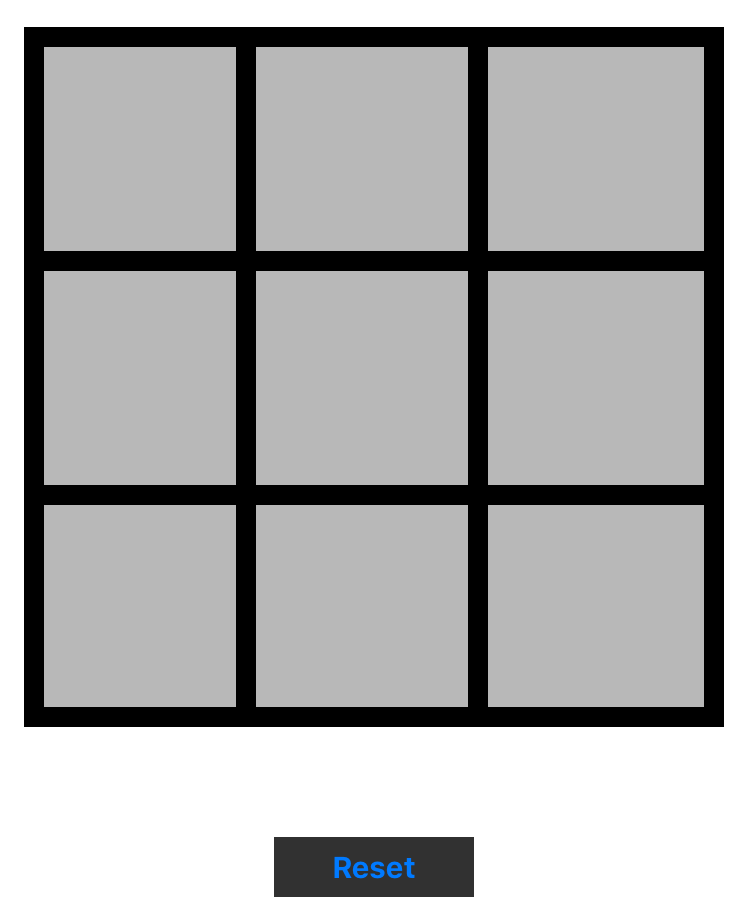
4th Screen
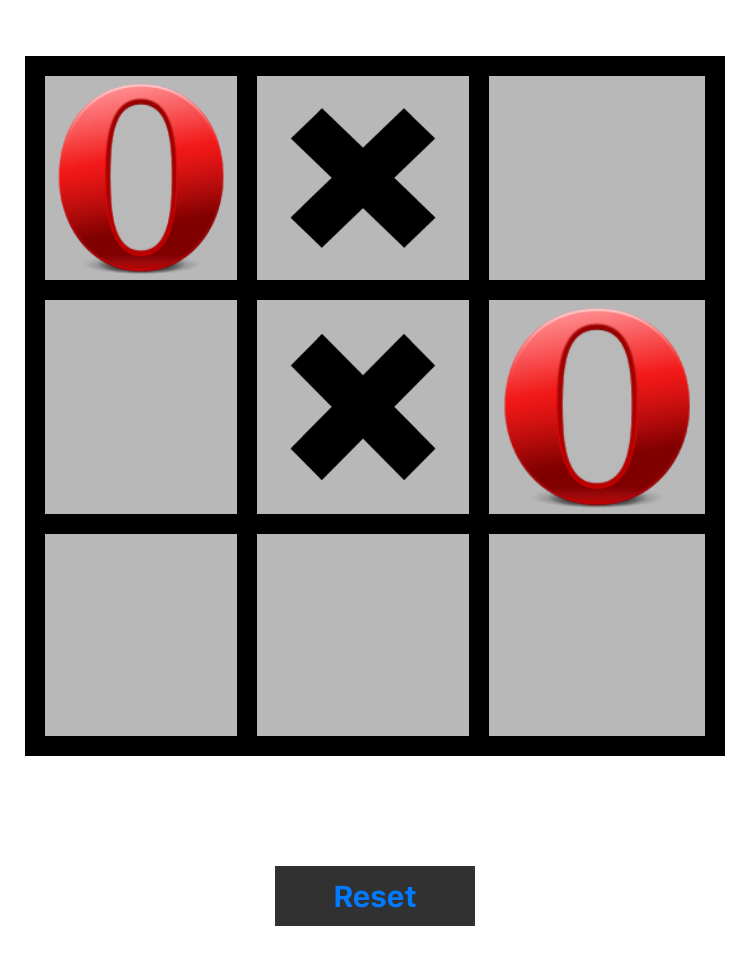
5th Screen
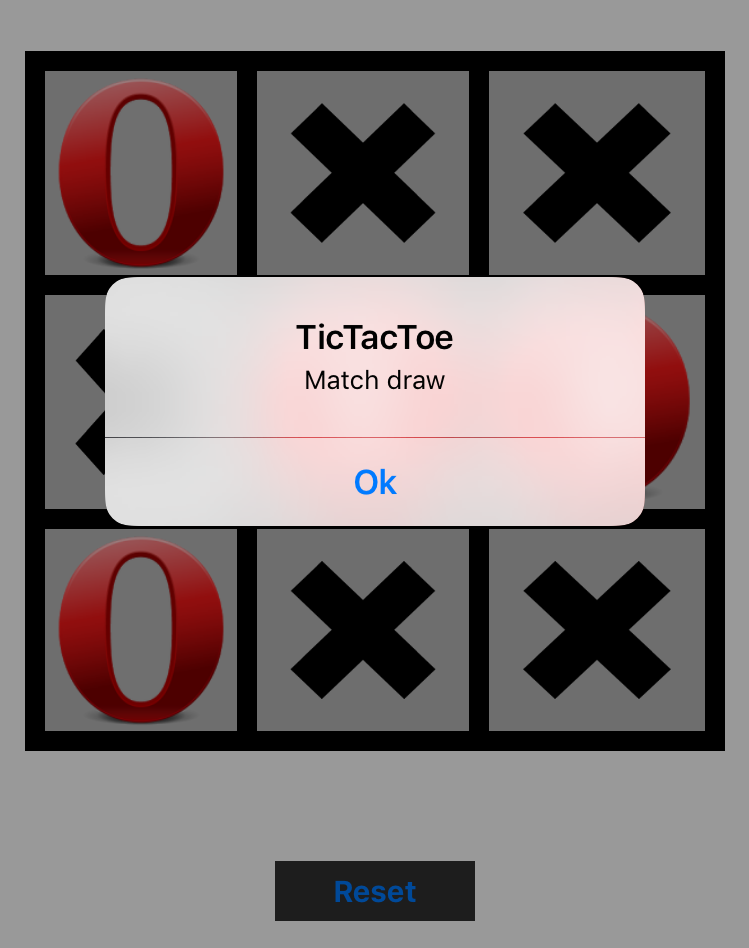
6th Screen
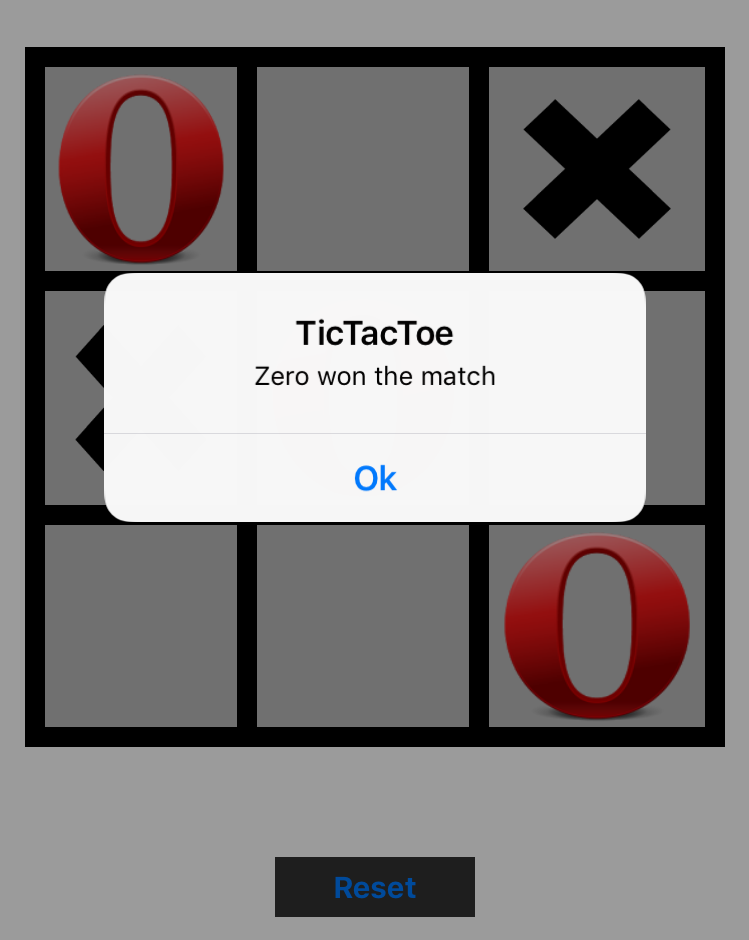
7th Screen
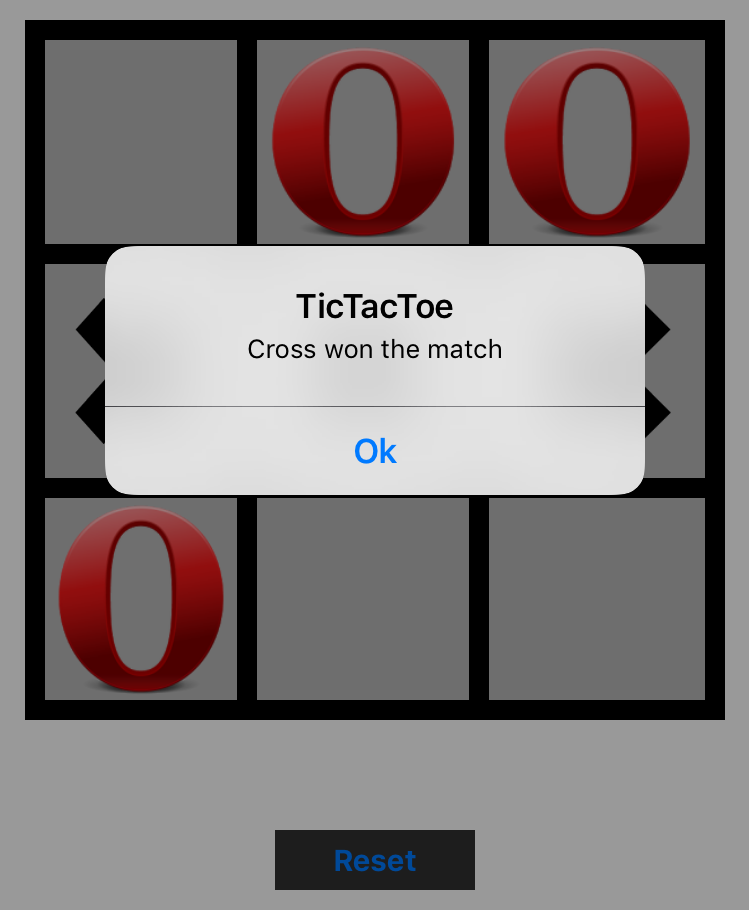
You can also download the sample code from below attached file. If you have any question feel free to post in comments
0 Comment(s)