Calling Web API from MVC Controller in ASP.NET
This blog defines calling of web api from mvc layer project. The following steps are to be followed :-
Step 1 : Create an empty solution in visual studio 2015. From file menu click New and then New Project. On left side of dialog box, from the menu, selection of Installed -> Templates -> Other Project Types -> select Visual Studio Solutions from the expanded list. In the middle pane select Blank solution give a name "WebApiWithMvc" and location for this solution.
Step 2 : Create two projects within "WebApiWithMvc" solution. First is an Mvc Project named "MvcApp" and second is a WebApi project named "WebApi". A default structure for both projects appear.
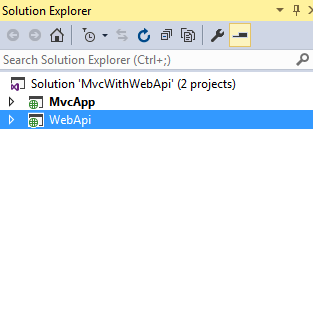
Step 3 : Add a model named "StudentModel" in Models folder of "WebApi" project.
namespace WebApi.Models
{
public class StudentModel
{
public int StudentId { get; set; }
public string StuName { get; set; }
}
}
Step 4 : Create Student data in action method (Get) which is within "valuescontroller" in Controllers folder of "WebApi" project. Below is the Get action method in "WebApi" project.
namespace WebApi.Controllers
{
public class ValuesController : ApiController
{
// GET api/values
public IEnumerable<StudentModel> Get()
{
List<StudentModel> list = new List<StudentModel>();
for (int i = 0; i < 10; i++)
{
StudentModel stu = new StudentModel();
stu.StudentId = i;
stu.StuName = "stu" + i;
list.Add(stu);
}
return list;
}
}
}
Step 5 : When this WebApi project runs following screen appears.
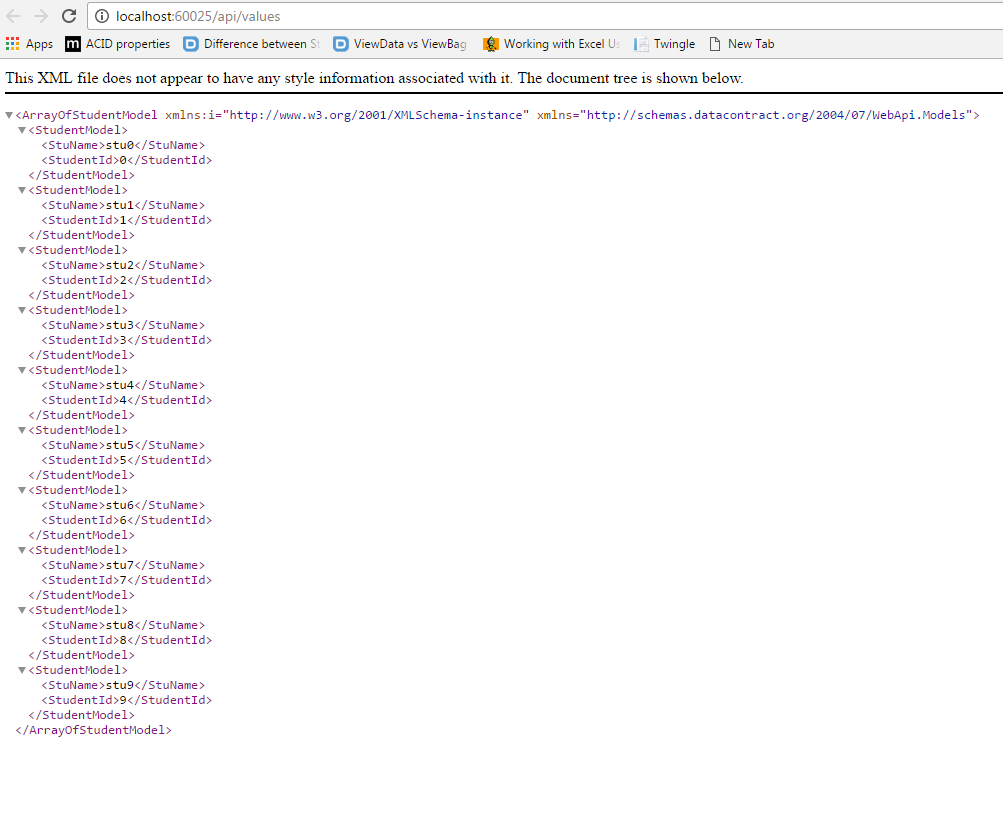
Step 6 : Now start working with Mvc project "MvcApp". Add the following key in Web.config file of "MvcApp" project.
<add key="baseurl" value="http://localhost:60025" />
Step 7 : Add a model named "StudentModel" in Models folder of "MvcApp" project.
namespace MvcApp.Models
{
public class StudentModel
{
public int StudentId { get; set; }
public string StuName { get; set; }
}
}
Step 8 : For accessing student data from "WebApi" project within "HomeController" in Controllers folder of "MvcApp" project add an action method named "Student_Detail".
using System;
using System.Collections.Generic;
using System.Web.Mvc;
using System.Net.Http;
using System.Configuration;
using System.Net.Http.Headers;
using MvcApp.Models;
using Newtonsoft.Json;
using System.Threading.Tasks;
namespace MvcApp.Controllers
{
public class HomeController : Controller
{
string apiUrl = ConfigurationManager.AppSettings["baseurl"] + "/api/Values/";
HttpClient client;
public HomeController()
{
client = new HttpClient();
client.BaseAddress = new Uri(apiUrl);
client.DefaultRequestHeaders.Accept.Clear();
client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
}
public async Task<ActionResult> Student_Detail()
{
List<StudentModel> emp = new List<StudentModel>();
HttpResponseMessage responseMessage = await client.GetAsync(apiUrl);
if (responseMessage.IsSuccessStatusCode)
{
var responseData = responseMessage.Content.ReadAsStringAsync().Result;
emp = JsonConvert.DeserializeObject<List<StudentModel>>(responseData);
}
return View(emp);
}
}
}
Step 9 : View against action method "Student_Detail".
@model IEnumerable<MvcApp.Models.StudentModel>
<table class="table">
<tr>
<th>
@Html.DisplayNameFor(model => model.StudentId)
</th>
<th>
@Html.DisplayNameFor(model => model.StuName)
</th>
<th></th>
</tr>
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.StudentId)
</td>
<td>
@Html.DisplayFor(modelItem => item.StuName)
</td>
</tr>
}
</table>
When above "MvcApp" runs by hitting Home.Student_Detail following screen appears
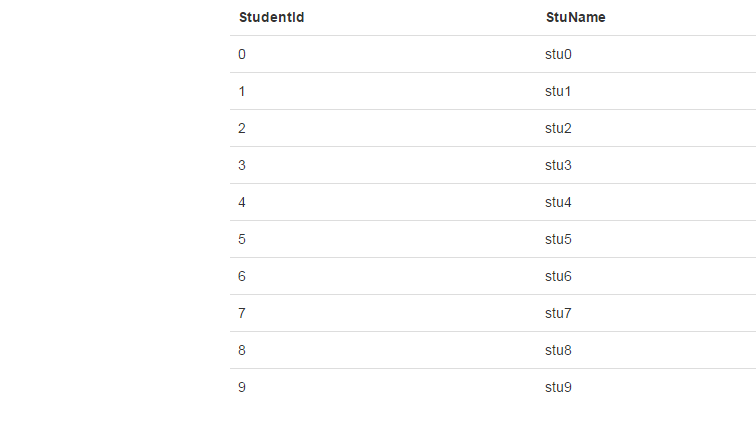
1 Comment(s)